Top 29 Algorithm Developer Interview Questions and Answers [Updated 2025]
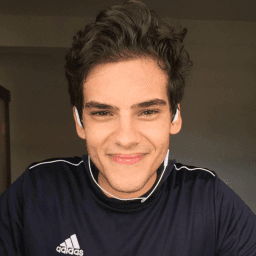
Andre Mendes
•
March 30, 2025
Navigating the interview process for an Algorithm Developer role can be daunting, but preparation is key to success. In this post, we provide a comprehensive collection of the most common interview questions you're likely to encounter, complete with example answers and effective tips. Whether you're a seasoned pro or just starting out, this guide will equip you with the insights needed to impress your interviewers and secure your dream job.
Download Algorithm Developer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Algorithm Developerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Algorithm Developer Interview Questions
Behavioral Interview Questions
Can you describe a challenging algorithmic problem you solved in the past and how you approached it?
How to Answer
- 1
Select a specific algorithmic problem you faced in a project.
- 2
Explain the context and the challenge briefly.
- 3
Describe the approach you took to solve it step by step.
- 4
Highlight the algorithm or technique used.
- 5
Discuss the outcome and what you learned from the experience.
Example Answers
In a previous project, I had to optimize a search algorithm in a large dataset. I identified that a binary search would be efficient given the data was sorted. I implemented it, reducing the search time from O(n) to O(log n). The project outcome improved performance significantly, validating the importance of selecting the right algorithm.
Tell me about a time you worked on an algorithm development project with a team. What was your role, and how did you contribute?
How to Answer
- 1
Choose a specific project to discuss.
- 2
Describe your role clearly and how you collaborated.
- 3
Highlight the skills or techniques you used in developing the algorithm.
- 4
Mention any challenges and how you helped to overcome them.
- 5
Conclude with the impact of your work on the project outcome.
Example Answers
In a team project to develop a real-time recommendation system, I was responsible for the collaborative design of the algorithm. I used machine learning techniques to analyze user data and improve suggestions. We faced data quality issues, so I led efforts to clean the dataset, which enhanced our model's accuracy and user satisfaction.
Don't Just Read Algorithm Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Algorithm Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Describe an occasion when you had to lead a group to develop a solution to a complex problem. How did you manage and coordinate the effort?
How to Answer
- 1
Identify a specific project or challenge you led.
- 2
Outline the problem clearly and the goals of the team.
- 3
Describe your leadership approach and how you motivated the team.
- 4
Discuss the tools or strategies you used to coordinate efforts.
- 5
Mention the outcome and any lessons learned.
Example Answers
In my last project, I led a team of five to develop an optimization algorithm for a logistics problem. We started by clearly defining our objectives and breaking down tasks. I held regular check-ins to ensure everyone was aligned and used project management software to track progress. Ultimately, we improved delivery times by 20%, and I learned the importance of clear communication.
Have you ever had a disagreement with a peer over the design of an algorithm? How did you handle it?
How to Answer
- 1
Identify the specific disagreement and your perspective on it.
- 2
Explain your approach to discussing the issue with your peer.
- 3
Highlight any steps taken to find common ground or a compromise.
- 4
Emphasize the importance of collaboration and learning from the situation.
- 5
Conclude with the outcome and what you learned from the experience.
Example Answers
In a recent project, my peer and I disagreed on the efficiency of a sorting algorithm. I initiated a discussion where we both presented our reasoning. After reviewing the trade-offs together, we decided to prototype both algorithms. This helped us find a middle ground and eventually led to a more optimized solution. I learned the value of collaboration.
Can you give an example of a time when you had to learn a new algorithmic concept quickly to complete a project?
How to Answer
- 1
Choose a specific example from your experience.
- 2
Explain the context of the project briefly.
- 3
Describe how you learned the algorithm quickly.
- 4
Mention the impact of using that algorithm on the project.
- 5
Highlight any challenges you overcame in the process.
Example Answers
In a recent project, I had to implement the Dijkstra algorithm for shortest path finding. The project required it quickly, so I spent a day reviewing the algorithm and implementing it using online resources. By doing this, I was able to optimize our route planning feature, which improved performance by 30%.
How do you stay updated with the latest trends and advancements in algorithm development?
How to Answer
- 1
Follow leading algorithm researchers on platforms like Twitter and LinkedIn
- 2
Subscribe to relevant journals and conferences in computer science
- 3
Engage in online courses or webinars focused on algorithm advancements
- 4
Participate in coding competitions and hackathons to practice new techniques
- 5
Join online communities or forums dedicated to algorithms and data structures
Example Answers
I follow key researchers on Twitter to keep up with their published work and insights. I also read papers from top conferences like NeurIPS and ICML regularly.
Describe a situation where you took the initiative to solve an unexpected problem in an algorithm development project.
How to Answer
- 1
Identify a specific unexpected problem you faced in a project.
- 2
Explain the steps you took to address the problem.
- 3
Highlight the impact of your initiative on the project outcome.
- 4
Discuss any skills or tools you used to solve the problem.
- 5
Conclude with a reflection on what you learned from the experience.
Example Answers
In my last project, we discovered that our algorithm was not scaling well with large datasets, which was unforeseen. I initiated an analysis to pinpoint the bottlenecks, implemented caching strategies, and refactored the algorithm. This reduced processing time by 40% and allowed us to meet project deadlines. I learned the importance of performance testing early in the development process.
Can you provide an example of how you managed your time and resources to meet a tight deadline in an algorithm development context?
How to Answer
- 1
Identify a specific project with a tight deadline.
- 2
Explain the algorithm context and what made it challenging.
- 3
Discuss how you prioritized tasks and allocated resources.
- 4
Mention any tools or techniques you used to stay on schedule.
- 5
Share the outcome and what you learned from the experience.
Example Answers
In my last project, we had to improve a sorting algorithm for a client within a week. I quickly assessed the existing code, identified bottlenecks, and prioritized optimizations. Using profiling tools, I focused on the most time-consuming parts, which helped reduce computation time significantly. We met the deadline, and the new algorithm was 30% faster.
Tell us about a time you received critical feedback on your algorithm design. How did you respond?
How to Answer
- 1
Be specific about the feedback you received.
- 2
Explain the context of your algorithm design project.
- 3
Describe your initial reaction and how you processed the feedback.
- 4
Highlight the changes you made based on the feedback.
- 5
Conclude with the positive outcome or what you learned.
Example Answers
In a recent project, my team lead pointed out that my sorting algorithm was not optimal for large datasets. I felt surprised, but I took the feedback seriously. I analyzed the algorithm's time complexity and researched more efficient methods. I refactored the code using a more advanced sorting technique, which improved performance by 30%. This experience taught me the value of seeking and accepting constructive criticism.
Technical Interview Questions
What are the core data structures you commonly use when developing algorithms, and when is it appropriate to use each?
How to Answer
- 1
Identify key data structures like arrays, linked lists, stacks, queues, trees, and hash tables.
- 2
Explain the performance characteristics of each data structure.
- 3
Discuss scenarios where each data structure excels, such as search or insertion efficiency.
- 4
Mention trade-offs involved in choosing different data structures.
- 5
Relate your answer to your personal experience or specific projects.
Example Answers
I often use arrays for situations where I need fast access to elements by index, such as when implementing simple sorting algorithms. For linked lists, they're useful for dynamic memory allocation when the size of the data set can't be determined in advance.
How do you analyze the time and space complexity of an algorithm?
How to Answer
- 1
Identify the basic operations that determine the algorithm's runtime.
- 2
Count how many times these operations are executed as the input size increases.
- 3
Use Big O notation to express the upper bound of the time complexity.
- 4
For space complexity, analyze the additional memory used relative to input size.
- 5
Consider the best, average, and worst case scenarios for a complete analysis.
Example Answers
To analyze time complexity, I look at the main operations in the algorithm, count how often they run based on input size, and express this in Big O notation. For instance, a loop running 'n' times results in O(n).
Don't Just Read Algorithm Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Algorithm Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Explain the concept of dynamic programming and provide an example where this approach is beneficial.
How to Answer
- 1
Define dynamic programming clearly and succinctly
- 2
Explain the problem-solving process used in dynamic programming
- 3
Provide a specific example of a problem that uses dynamic programming
- 4
Highlight the benefits of using dynamic programming over brute force methods
- 5
Conclude with a brief summary of when to use this technique
Example Answers
Dynamic programming is a method used to solve problems by breaking them down into smaller subproblems and storing the results to avoid redundant calculations. A common example is the Fibonacci sequence, where using dynamic programming we can calculate Fibonacci numbers in linear time instead of exponential time using recursion.
What algorithms would you use to solve a shortest path problem in a weighted graph?
How to Answer
- 1
Identify the type of graph: directed or undirected, weighted or unweighted.
- 2
Consider the nature of the weights: are they all positive or can they be negative?
- 3
For graphs with non-negative weights, recommend Dijkstra's algorithm.
- 4
If negative weights are possible, suggest the Bellman-Ford algorithm.
- 5
Highlight the A* algorithm for shortest paths with heuristics.
Example Answers
For a weighted graph with non-negative weights, I would use Dijkstra's algorithm. It efficiently finds the shortest path from a source to all other nodes.
Explain how you would approach optimizing an existing algorithm for better performance.
How to Answer
- 1
Identify the current time and space complexity of the algorithm.
- 2
Profile the algorithm to find bottlenecks in performance.
- 3
Explore algorithmic improvements, like dynamic programming or divide and conquer.
- 4
Consider data structure changes that can enhance performance.
- 5
Test your optimized algorithm to ensure it maintains correctness.
Example Answers
First, I would analyze the current algorithm to determine its time and space complexity. Then, I'd use profiling tools to pinpoint slow sections. After that, I'd consider applying dynamic programming if there are overlapping subproblems. Next, I'd check if a different data structure, like a hash table, could improve lookup times. Finally, I would thoroughly test the new implementation to confirm it works as intended.
How do algorithm development and machine learning intersect, and what specific skills are necessary for working at this intersection?
How to Answer
- 1
Define algorithm development and machine learning
- 2
Explain their relationship with clear examples
- 3
Identify key skills like programming, statistics, and data analysis
- 4
Mention knowledge in optimization techniques and model evaluation
- 5
Highlight the importance of continuous learning in both areas
Example Answers
Algorithm development focuses on creating efficient methods for problem-solving, while machine learning involves training models to learn patterns from data. They intersect as machine learning relies on algorithms for tasks like classification and regression. Skills needed include proficiency in programming languages like Python, a solid understanding of statistics, and experience with data manipulation and analysis.
What are the strategies you consider when adapting an algorithm for parallel execution?
How to Answer
- 1
Identify independent tasks that can run concurrently
- 2
Use data partitioning to distribute workload among processors
- 3
Minimize communication overhead between parallel tasks
- 4
Ensure tasks are balanced to avoid idle processors
- 5
Utilize parallel programming libraries or frameworks for implementation
Example Answers
First, I look for independent tasks that can be executed simultaneously. Then I partition the data effectively to distribute it among the available processors, while keeping the communication between tasks to a minimum.
How do you ensure that the algorithms you develop are secure against potential vulnerabilities?
How to Answer
- 1
Conduct regular code reviews and static analysis to catch security flaws early
- 2
Follow industry best practices such as OWASP when developing algorithms
- 3
Implement input validation to prevent injection attacks and ensure data integrity
- 4
Keep up to date with the latest security research and vulnerabilities relevant to your algorithms
- 5
Plan for threat modeling to identify potential risks during the design phase
Example Answers
I ensure algorithm security by conducting thorough code reviews and using static analysis tools to identify vulnerabilities. I regularly follow OWASP guidelines for secure coding practices and always implement strict input validation to prevent any kind of injection attacks.
Describe the process you typically follow when designing a new algorithm.
How to Answer
- 1
Start by clearly defining the problem you are solving.
- 2
Research existing solutions and understand their strengths and weaknesses.
- 3
Outline your high-level approach before diving into details.
- 4
Consider edge cases and constraints that may affect your algorithm.
- 5
Iterate on your design, optimizing for efficiency and clarity.
Example Answers
I begin by defining the problem clearly, then I research current solutions to identify gaps. I outline my approach, focusing on edge cases, and iterate until I achieve an efficient algorithm.
What methods do you use to test and validate the correctness of an algorithm?
How to Answer
- 1
Define the expected outputs for known inputs clearly.
- 2
Use unit tests to check individual components of the algorithm.
- 3
Perform boundary testing to examine edge cases.
- 4
Analyze the algorithm's complexity and performance in addition to correctness.
- 5
Use assertions in code to ensure invariants hold true during execution.
Example Answers
I create a set of test cases with known outcomes and use unit tests to validate each part of the algorithm. I also check edge cases like empty inputs or maximum values to ensure robustness.
Don't Just Read Algorithm Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Algorithm Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Situational Interview Questions
You are tasked with improving a legacy algorithm that is critical to the company's product. How would you approach this task?
How to Answer
- 1
Analyze the current algorithm to understand its strengths and weaknesses.
- 2
Gather performance metrics and identify bottlenecks or inefficiencies.
- 3
Consult with team members or stakeholders to gather insights on the algorithm's limitations.
- 4
Consider modern approaches or technologies that could enhance the algorithm.
- 5
Implement changes incrementally and test rigorously to ensure stability and performance.
Example Answers
First, I would analyze the existing algorithm to pinpoint its weaknesses. Then, I would collect performance data to identify specific bottlenecks. It's also important to discuss with the team to understand user concerns. Next, I would explore new techniques or optimizations, like parallel processing. Finally, I would implement changes in small steps, testing each modification for performance gains.
Imagine you need to scale an algorithm originally designed for a small dataset to handle millions of entries. What changes would you consider?
How to Answer
- 1
Analyze the time and space complexity of the current algorithm.
- 2
Consider optimizations such as data structures that provide faster access times.
- 3
Implement parallel processing or distributed computing if applicable.
- 4
Explore data partitioning strategies to handle large datasets more efficiently.
- 5
Utilize caching to avoid repeated computations on the same data.
Example Answers
I would first analyze the algorithm's complexity to identify bottlenecks. For instance, if it uses O(n^2) time, I'll consider switching to a more efficient data structure like a hash table for faster access.
Don't Just Read Algorithm Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Algorithm Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Given the requirement to develop a real-time search functionality for massive datasets, which algorithms would you evaluate?
How to Answer
- 1
Consider the types of queries that will be made on the datasets
- 2
Evaluate the trade-offs between search speed and accuracy
- 3
Explore indexing techniques like inverted indexes or tries
- 4
Assess the need for distributed search frameworks for scalability
- 5
Mention any relevant algorithms like Aho-Corasick or Elasticsearch for implementation
Example Answers
For real-time search, I'd evaluate using inverted indexes to quickly find documents containing specific keywords. I'd also look into using Elasticsearch, as it offers distributed search capabilities and can handle massive datasets efficiently.
You discover a bug in an algorithm after deployment that intermittently causes errors. How would you proceed to identify and resolve the issue?
How to Answer
- 1
Reproduce the bug using test cases that reflect real user scenarios
- 2
Examine the logs and error messages for clues about the bug
- 3
Isolate parts of the algorithm to narrow down where the issue might be
- 4
Consult with team members or use code reviews to gain new insights
- 5
Develop a fix and thoroughly test it before redeploying
Example Answers
I would first try to reproduce the bug under controlled conditions to see when it occurs. Next, I'd look into the relevant logs to identify any patterns or specific error messages that occur during the failures. Once I narrow it down, I would isolate sections of the algorithm to figure out where the bug lies. Collaboration with my team would help, as sometimes discussing the problem can reveal new perspectives. Finally, after identifying and fixing the bug, I'd ensure comprehensive testing before redeployment.
Your team is considering different technologies for implementing a complex machine learning algorithm. How would you evaluate and decide on the best technology?
How to Answer
- 1
Identify the specific requirements of the algorithm and the project goals
- 2
Research and compare the performance benchmarks of each technology
- 3
Consider the ease of integration with existing systems and tools
- 4
Assess the support and community around each technology
- 5
Evaluate the scalability and future-proofing of the technology choices
Example Answers
I would start by clarifying the algorithm's requirements and how it aligns with our project goals. Then, I'd compare the technologies based on performance metrics and ease of integration with our tools. I'd also look into community support available and how well each can scale.
You receive conflicting requirements from a client regarding algorithm functionality. How would you handle this situation?
How to Answer
- 1
Clarify the requirements by asking specific questions.
- 2
Identify the priorities and needs of the client.
- 3
Document everything to refer back to later.
- 4
Suggest a meeting to discuss conflicts openly.
- 5
Propose a solution that accommodates both requirements, if possible.
Example Answers
I would first arrange a meeting with the client to clarify the conflicting requirements and understand their priorities. Taking notes would help keep track of the discussion for future reference.
An algorithm you're developing may unfairly disadvantage a certain group of users. How would you address this issue?
How to Answer
- 1
Identify and analyze the biased outcomes of the algorithm
- 2
Engage with affected users to understand their experiences
- 3
Adjust the algorithm to mitigate biases, such as re-weighting features
- 4
Implement fairness metrics to monitor the algorithm's performance
- 5
Ensure transparency and documentation of the algorithm's decisions
Example Answers
I would first analyze the data to identify any biased outcomes. Then, I would engage with affected users to capture their perspectives. Afterward, I'd modify the algorithm to reduce bias, possibly by re-weighting certain features. Lastly, I'd establish fairness metrics to monitor how well the algorithm performs across different groups.
You need to deliver a complex algorithm under tight deadlines. How would you ensure the quality of your work?
How to Answer
- 1
Break the algorithm down into smaller, manageable components for easier tackling.
- 2
Write unit tests for each component as you develop them to catch issues early.
- 3
Conduct regular code reviews with peers to gather immediate feedback.
- 4
Prioritize key functionalities and ensure they work before optimizing the algorithm.
- 5
Document your code and thought process to facilitate debugging and future improvements.
Example Answers
I would begin by breaking the algorithm into smaller tasks and focusing on one at a time. By writing unit tests for each part as I go, I can catch any errors early on. I would also schedule quick code reviews with colleagues to get feedback. Once the critical parts are working, I would then optimize.
How would you approach the development of an innovative algorithm for a new application domain?
How to Answer
- 1
Identify the specific needs and problems of the application domain
- 2
Research existing algorithms and techniques in similar domains
- 3
Brainstorm potential new approaches or modifications to existing algorithms
- 4
Prototype and test the proposed algorithm with real-world data
- 5
Iterate on the algorithm based on feedback and performance metrics
Example Answers
First, I would analyze the specific challenges faced by the application domain to understand user needs. Next, I'd review existing algorithms in related fields to gather insights. From there, I would brainstorm innovative modifications that could enhance these algorithms. After that, I would prototype an initial version and test it with real data. Finally, I would refine the algorithm based on the testing results.
You are collaborating with a cross-functional team where members have different understanding of algorithms. How would you effectively communicate your ideas?
How to Answer
- 1
Assess the team's expertise level before communicating
- 2
Use analogies and simple terms to explain complex concepts
- 3
Encourage questions to gauge understanding and clarity
- 4
Utilize visual aids like diagrams or flowcharts to illustrate ideas
- 5
Be patient and open to feedback, adapting your explanations as needed
Example Answers
I would start by assessing the team's background and tailor my explanations to their level. I might use analogies like comparing algorithms to recipes to explain how they work. Encouraging questions helps ensure everyone is on the same page.
Don't Just Read Algorithm Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Algorithm Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Algorithm Developer Position Details
Recommended Job Boards
CareerBuilder
www.careerbuilder.com/jobs/algorithm-developerZipRecruiter
www.ziprecruiter.com/Jobs/Algorithm-DeveloperThese job boards are ranked by relevance for this position.
Related Positions
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates