Top 30 Java Software Engineer Interview Questions and Answers [Updated 2025]
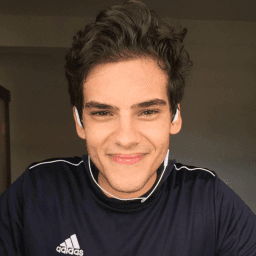
Andre Mendes
•
March 30, 2025
Preparing for a Java Software Engineer interview can be daunting, but our updated guide for 2025 is here to help. Discover the most common interview questions for this role, complete with example answers and insightful tips to help you respond confidently and effectively. Whether you're a seasoned developer or a newcomer, this post is designed to sharpen your skills and boost your interview performance.
Download Java Software Engineer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Java Software Engineerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Java Software Engineer Interview Questions
Behavioral Interview Questions
Can you describe a time when you successfully worked on a project with a diverse team, and what was your role in it?
How to Answer
- 1
Start by briefly describing the project and its goals.
- 2
Explain the diversity of the team and why it mattered.
- 3
Detail your specific role and contributions to the team.
- 4
Highlight how you collaborated and communicated effectively.
- 5
Reflect on the outcomes and what you learned from the experience.
Example Answers
In my last position, I worked on a mobile app project that aimed to enhance user accessibility. Our team included members from different backgrounds, including web developers and UX designers. As a Java developer, I focused on backend integration while working closely with the designers to ensure our APIs supported their needs. Regular meetings and collaborative tools helped us align our goals. The app launched successfully, and we received positive feedback on its inclusive features.
Tell us about a challenging bug you faced in a Java application and how you resolved it.
How to Answer
- 1
Start with the context of the application and the bug.
- 2
Explain the impact the bug had on the application or users.
- 3
Describe the steps you took to diagnose the issue.
- 4
Mention any tools or methods you used for debugging.
- 5
Conclude with the solution and what you learned from the experience.
Example Answers
In a previous project, I was working on a data processing application. We encountered a bug where the application would occasionally throw NullPointerExceptions. This was critical as it affected data integrity. I used logging to trace where the null values came from, and discovered it was due to an uninitialized variable in a complex logic branch. After fixing the initialization issue, I also improved the error handling to prevent similar bugs.
Don't Just Read Java Software Engineer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Software Engineer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Have you ever had a conflict with a coworker regarding code implementation? How did you handle it?
How to Answer
- 1
Stay calm and professional when discussing the conflict
- 2
Clearly state the different viewpoints without assigning blame
- 3
Focus on the goal of producing the best solution for the project
- 4
Suggest a collaborative approach to resolve the disagreement
- 5
Highlight any specific outcomes or lessons learned from the experience
Example Answers
Yes, I had a disagreement about using a certain framework. I calmly explained my perspective and listened to my coworker's views. We decided to prototype both solutions to see which one performed better, which helped us pick the right approach together.
Describe a time when you had to take the lead on a team project. What was your approach and what was the outcome?
How to Answer
- 1
Share a specific project where you led the team
- 2
Explain your leadership style and decision-making process
- 3
Highlight challenges faced and how you overcame them
- 4
Discuss the results achieved and any metrics if applicable
- 5
Reflect on what you learned from the experience
Example Answers
In a recent project to develop a new feature for our application, I took the lead. I organized our meetings, set clear goals, and delegated tasks based on team members' strengths. We faced a tight deadline, but by prioritizing our tasks and using agile methods, we completed the feature on time and received positive feedback from users, increasing engagement by 20%. I learned the importance of clear communication and adaptability.
Technical Interview Questions
Explain the differences between an interface and an abstract class in Java.
How to Answer
- 1
Define what an interface is and what an abstract class is.
- 2
Highlight key differences in terms of method implementations.
- 3
Explain how inheritance works for each and their use cases.
- 4
Mention the limitations of each, such as multiple inheritance for interfaces.
- 5
Use an example to illustrate your point.
Example Answers
An interface in Java is a contract that defines methods without implementations, while an abstract class can provide some method implementations. Interfaces allow for multiple inheritance, whereas a class can only extend one abstract class. You would use interfaces for defining capabilities, while abstract classes are best for sharing code among related classes.
What are the four main principles of Object-Oriented Programming, and how are they implemented in Java?
How to Answer
- 1
Define each principle clearly: Encapsulation, Inheritance, Polymorphism, Abstraction.
- 2
Give a brief Java code example for each principle to illustrate your points.
- 3
Use real-world analogies when explaining concepts for better understanding.
- 4
Mention how these principles improve code maintainability and reusability.
- 5
Practice explaining these concepts succinctly to avoid long-winded answers.
Example Answers
The four main principles of Object-Oriented Programming are Encapsulation, Inheritance, Polymorphism, and Abstraction. In Java, encapsulation is implemented using access modifiers like private and public, which control access to class members. Inheritance allows a class to inherit fields and methods from another class using the extends keyword. Polymorphism is achieved through method overriding and interfaces. Abstraction hides complex implementation details, typically via abstract classes or interfaces.
Don't Just Read Java Software Engineer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Software Engineer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
How would you implement a stack using Java? Can you write a basic example?
How to Answer
- 1
Define a class for the stack and use an ArrayList or an array.
- 2
Implement at least two key methods: push() and pop().
- 3
Ensure you handle underflow when popping from an empty stack.
- 4
Consider implementing a size() method to track the number of elements.
- 5
Write a clear main method to demonstrate usage of your stack.
Example Answers
I would create a Stack class with an ArrayList to hold the elements. The push method adds an element to the end of the list, and pop removes the last element, checking if the list is empty first.
What is the difference between a thread and a process in Java, and how do you create threads in Java?
How to Answer
- 1
Define a process as an independent program with its own memory and resources.
- 2
Explain a thread as a lightweight process that shares resources with other threads in the same process.
- 3
Mention that a process can contain multiple threads for parallel execution.
- 4
Discuss creating threads using the Thread class or by implementing the Runnable interface.
- 5
Provide a simple code snippet example for thread creation.
Example Answers
A process is an independent execution unit with its own memory while a thread is a lightweight execution unit that shares the process's memory. You can create a thread by extending the Thread class or implementing the Runnable interface, like so: `new Thread(() -> { /* task */ }).start();`
What are the main differences between ArrayList and LinkedList?
How to Answer
- 1
Focus on key properties of each data structure
- 2
Mention performance characteristics for common operations
- 3
Highlight use cases for each type
- 4
Discuss memory usage differences
- 5
Be prepared to explain why one might be preferred over the other
Example Answers
ArrayList uses a dynamic array, while LinkedList uses nodes and links to connect elements. ArrayList has faster access time for getting elements, but LinkedList is better for adding/removing elements at the start or end.
How does exception handling work in Java? Can you give an example using try-catch blocks?
How to Answer
- 1
Explain the basics of exceptions in Java and the purpose of exception handling.
- 2
Define the try, catch, and finally blocks briefly.
- 3
Use a clear example showing how to handle a specific exception, such as ArithmeticException or NullPointerException.
- 4
Mention the importance of checking for exceptions and ensuring the program doesn't crash.
- 5
Conclude by noting that exception handling promotes cleaner code and better user experience.
Example Answers
In Java, exception handling allows us to manage runtime errors, preventing the program from crashing. We use try-catch blocks to catch exceptions. For example, we can catch an ArithmeticException like this: try { int result = 10 / 0; } catch (ArithmeticException e) { System.out.println('Cannot divide by zero.'); }
What are some key features of the Java Standard Library that you frequently use?
How to Answer
- 1
Identify commonly used packages like java.util and java.io.
- 2
Mention specific classes such as ArrayList, HashMap, or File.
- 3
Explain how you use these features in your projects.
- 4
Relate features to solving real-world problems.
- 5
Keep your answer concise and focused on practical use cases.
Example Answers
I frequently use the java.util package, especially classes like ArrayList for dynamic arrays and HashMap for key-value storage. In my recent project, I used ArrayList to manage a collection of user inputs efficiently.
What is Spring Framework and what are its core concepts and features?
How to Answer
- 1
Start by defining Spring Framework clearly.
- 2
Mention its purpose in building Java applications.
- 3
Discuss core concepts like Dependency Injection and Aspect-Oriented Programming.
- 4
Highlight key features such as Spring MVC, Spring Boot, and Spring Data.
- 5
Conclude with comments on its popularity and community support.
Example Answers
Spring Framework is a powerful framework for building Java applications, aimed at simplifying development and promoting good design practices. Key concepts include Dependency Injection, which helps manage dependencies, and Aspect-Oriented Programming for cross-cutting concerns. Important features are Spring MVC for web applications, Spring Boot for easy project setup, and Spring Data for database access. It's widely adopted in the industry due to its flexibility and extensive community support.
How does JPA differ from Hibernate, and can you provide a basic example of mapping a Java object to a database table?
How to Answer
- 1
Focus on the key difference between JPA and Hibernate: JPA is a specification, Hibernate is an implementation.
- 2
Mention that Hibernate is a JPA provider and gives additional features beyond the JPA specification.
- 3
Explain the basic annotations used in JPA for mapping Java classes to database tables, like @Entity, @Id, and @Table.
- 4
Provide a simple Java class example and a corresponding database table description to illustrate the mapping.
- 5
Keep your explanation focused and concise, avoiding overly technical jargon.
Example Answers
JPA is a Java specification for object-relational mapping, while Hibernate is an implementation of JPA that provides additional features. For example, we can use @Entity to map a Java class to a database table. Here’s a basic example:
Can you explain the Singleton design pattern and its use case in Java?
How to Answer
- 1
Define the Singleton pattern simply and directly.
- 2
Explain its purpose: ensuring a class has only one instance.
- 3
Mention how it is implemented in Java, particularly the use of a private constructor.
- 4
Discuss thread safety and lazy initialization briefly.
- 5
Provide a practical use case where it is beneficial.
Example Answers
The Singleton pattern ensures a class has only one instance. In Java, this is achieved by using a private constructor and a static method that returns the instance. It's often used for logging or managing shared resources, like a connection pool.
Don't Just Read Java Software Engineer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Software Engineer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What tools do you use for unit testing Java applications and how do you ensure coverage?
How to Answer
- 1
Mention specific tools like JUnit and Mockito for unit testing.
- 2
Explain how you write tests to cover different scenarios.
- 3
Discuss the use of code coverage tools like JaCoCo or Cobertura.
- 4
Highlight the importance of continuous integration for running tests.
- 5
Emphasize the role of TDD in your development process.
Example Answers
I primarily use JUnit for unit testing and Mockito for mocking dependencies. I ensure coverage by writing tests for both normal and edge cases and use JaCoCo to measure coverage, aiming for at least 80%.
What techniques do you use for optimizing the performance of Java applications?
How to Answer
- 1
Profile the application to identify bottlenecks using tools like JVisualVM or JProfiler
- 2
Use appropriate data structures and algorithms to improve performance
- 3
Minimize object creation by reusing objects and using primitive types where possible
- 4
Optimize database queries and use caching to reduce latency
- 5
Consider using asynchronous processing to improve responsiveness
Example Answers
I profile the application with JVisualVM to find bottlenecks. After identifying slow parts, I optimize algorithms and data structures accordingly. For example, using ArrayLists instead of LinkedLists for frequent access speeds things up.
How does garbage collection work in Java and what are some strategies to manage memory usage?
How to Answer
- 1
Explain that garbage collection is automatic memory management in Java.
- 2
Mention that it identifies and discards objects no longer in use.
- 3
Discuss common algorithms like mark-and-sweep and generational collection.
- 4
Highlight the importance of minimizing object creation and using weak references.
- 5
Suggest profiling tools for monitoring memory usage.
Example Answers
Garbage collection in Java automatically manages memory by identifying objects that are no longer referenced. It primarily uses algorithms like mark-and-sweep and generational garbage collection to reclaim memory. To manage memory usage effectively, it's good to minimize object creation and utilize weak references where appropriate.
What security practices do you follow when developing Java applications?
How to Answer
- 1
Use secure coding practices to avoid common vulnerabilities like SQL injection and XSS.
- 2
Implement input validation and data sanitization to protect against malformed input.
- 3
Utilize Java's built-in security features, such as the SecurityManager and access controls.
- 4
Regularly update dependencies to mitigate known vulnerabilities.
- 5
Perform thorough testing, including security testing like penetration testing.
Example Answers
I always follow secure coding practices to prevent vulnerabilities like SQL injection, and I ensure that all user inputs are validated and sanitized before processing them.
What new features in the latest Java releases have you used, and how have they helped improve your code?
How to Answer
- 1
Identify specific Java versions and features you have used.
- 2
Explain how these features improved code readability or efficiency.
- 3
Provide real-world examples or scenarios from your projects.
- 4
Mention any impact on performance or developer productivity.
- 5
Stay current by showing awareness of the latest Java updates.
Example Answers
In Java 11, I utilized the var keyword for local variable type inference, which reduced boilerplate code and enhanced readability in my methods. For example, instead of specifying types in complex streams, I could write 'var stream = list.stream()' and focus more on business logic.
Situational Interview Questions
Suppose a production system is experiencing intermittent slowdowns. How would you approach diagnosing and resolving the issue?
How to Answer
- 1
Review system logs and metrics to pinpoint the times of slowdown
- 2
Check for resource bottlenecks such as CPU, memory, or disk I/O
- 3
Analyze application performance using profiling tools
- 4
Investigate recent changes or deployments that might have introduced the issue
- 5
Consider environmental factors like network latency or external service dependencies
Example Answers
I would start by analyzing system logs around the time the slowdowns occur to identify any error patterns. Then, I would check resource utilization metrics to see if any resources are being overused, such as CPU or memory. If needed, I'd use a profiling tool to get deeper insights into application performance.
Imagine you are halfway through a project when the client changes the requirements. How would you handle this situation?
How to Answer
- 1
Assess the impact of the new requirements on the project timeline and deliverables
- 2
Communicate with the client to understand their priorities and reasons for the change
- 3
Involve your team to evaluate the feasibility of the new requirements
- 4
Propose a revised plan that accommodates the changes while managing expectations
- 5
Document all changes and keep transparent communication throughout the process
Example Answers
I would first assess how the changes affect our timeline and resources. Then, I'd discuss with the client to understand their motivations. After that, I'd work with my team to determine what can realistically be done and propose a new plan with adjusted timelines.
Don't Just Read Java Software Engineer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Software Engineer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
You are asked to review a junior developer's code, and you find it suboptimal. How would you provide constructive feedback?
How to Answer
- 1
Start with positive aspects of the code to build rapport
- 2
Highlight specific issues and explain why they are suboptimal
- 3
Provide alternatives or solutions to improve the code
- 4
Encourage questions and discussion for clarity
- 5
Follow up with an offer for further help or pair programming
Example Answers
I would begin by acknowledging what the junior developer did well, then point out specific areas that could be improved, such as naming conventions or performance issues. I'd suggest alternatives and invite them to ask questions.
If you are given a high-priority task with a tight deadline that overlaps with your current workload, how would you manage your tasks?
How to Answer
- 1
Assess the urgency and importance of each task
- 2
Communicate with your team or manager about priorities
- 3
Break down the tasks into manageable steps
- 4
Focus on the high-priority task first
- 5
Use tools or techniques to track your progress
Example Answers
I would first assess both tasks to determine which one is more urgent and important. Then, I would communicate with my manager to clarify priorities. Once I understand what needs to be done, I would break the high-priority task into smaller steps and focus on completing it before returning to my other workload.
Your team can't agree on the best approach to solve a technical problem. How would you facilitate reaching a consensus?
How to Answer
- 1
Encourage open discussion by inviting each team member to share their perspective.
- 2
Identify the common goals and priorities of the team to focus the discussion.
- 3
Facilitate a pros and cons analysis of each proposed solution.
- 4
Use a voting method to gauge team support for the best ideas.
- 5
Summarize the outcomes and next steps to ensure clarity.
Example Answers
I would start by encouraging everyone to share their viewpoints on the problem. Then, I would highlight our common goals to steer the discussion. After that, we could list the pros and cons of each approach. Finally, I would suggest a vote to see which solution has the most support and summarize our action steps.
You've identified a significant amount of technical debt in a legacy system. How would you prioritize addressing it within ongoing projects?
How to Answer
- 1
Assess the impact of the technical debt on system performance and team productivity
- 2
Prioritize debt that blocks new feature development or causes frequent bugs
- 3
Engage with stakeholders to understand business priorities
- 4
Plan incremental refactoring as part of the sprint cycle
- 5
Document technical debt items clearly for transparency and future reference
Example Answers
I would start by evaluating which areas of the legacy system are causing the most issues in terms of performance and maintenance. I would then prioritize those that directly hinder new feature development, as those are critical for keeping the product competitive. Collaboration with the team and stakeholders would be key to align our efforts with business goals, and I would propose including technical debt tasks into our regular sprint planning.
Your company decides to adopt a new technology that you are not familiar with. How would you prepare yourself and your team for this change?
How to Answer
- 1
Research the new technology through official documentation and tutorials
- 2
Enroll in online courses or workshops to gain practical experience
- 3
Set up a team meeting to discuss the technology and share knowledge
- 4
Create a project plan to implement the new technology step-by-step
- 5
Encourage a culture of continuous learning and experimentation with the new tech
Example Answers
I would start by researching the new technology through its official documentation and online tutorials to understand the fundamentals. Next, I would suggest enrolling in a relevant online course for a deeper dive. I would then organize a team meeting where we can share our findings and plan our approach. Additionally, I would create a project plan to implement the technology gradually, allowing everyone to adapt comfortably.
A major client reports that their requirements are not being met by the current version of your software. How would you address their concerns?
How to Answer
- 1
Acknowledge the client's concerns and thank them for their feedback.
- 2
Schedule a meeting to discuss the specific requirements in detail.
- 3
Investigate the current software limitations related to their needs.
- 4
Propose a plan for addressing the gaps, including timelines and resources.
- 5
Communicate regularly during the resolution process to keep the client updated.
Example Answers
Thank you for bringing this to our attention. I’d like to meet with you to understand your specific requirements better and identify gaps in the current software. Once we clarify the issues, I will work on a plan to address them and keep you updated on our progress.
The application you are working on needs to scale to handle a 10x increase in load. What steps would you take to ensure performance and stability?
How to Answer
- 1
Analyze the current system architecture for bottlenecks.
- 2
Implement horizontal scaling by adding more instances or servers.
- 3
Utilize caching mechanisms to reduce database load.
- 4
Optimize database queries and consider using a read replica.
- 5
Monitor performance metrics in real-time to identify issues quickly.
Example Answers
To handle a 10x increase in load, I would first analyze the application architecture to find bottlenecks. Then, I would implement horizontal scaling by adding more server instances, along with caching frequent queries to reduce database load. I would also optimize any inefficient database queries and use read replicas. Finally, I would set up monitoring to keep track of performance metrics.
You're part of a cross-functional team including designers, PMs, and other engineers. How would you ensure effective collaboration and communication within the team?
How to Answer
- 1
Establish regular check-in meetings to discuss progress and issues
- 2
Use collaborative tools like Jira or Trello for transparency on tasks
- 3
Encourage open communication through chat platforms like Slack
- 4
Respect everyone’s expertise and involve them in decision-making
- 5
Document key decisions and processes to maintain clarity
Example Answers
I would set up weekly check-in meetings to align with the team on our goals. Using Jira, we can keep track of tasks and ensure everyone is updated on what's being worked on.
Don't Just Read Java Software Engineer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Software Engineer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Java Software Engineer Position Details
Recommended Job Boards
CareerBuilder
www.careerbuilder.com/jobs-java-software-engineerZipRecruiter
www.ziprecruiter.com/Jobs/Java-Software-EngineerThese job boards are ranked by relevance for this position.
Related Positions
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates