Top 30 C Developer Interview Questions and Answers [Updated 2025]
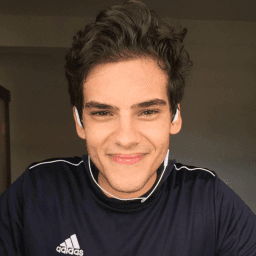
Andre Mendes
•
March 30, 2025
Navigating the competitive landscape of C Developer interviews requires a solid understanding of common questions and strategic responses. In this updated guide for 2025, we explore essential interview questions, providing example answers and valuable tips to help you respond effectively. Whether you're a seasoned programmer or just starting your career, this post is designed to equip you with the insights needed to impress potential employers.
Download C Developer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top C Developerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of C Developer Interview Questions
Technical Interview Questions
What tools and methodologies do you use to debug a C program?
How to Answer
- 1
Start with mentioning built-in debugging options like gdb.
- 2
Highlight preprocessor tools for code examination, like gcc with flags.
- 3
Discuss the importance of inserting print statements strategically.
- 4
Mention memory checkers like Valgrind to catch memory issues.
- 5
Explain the potential of using IDEs with integrated debugging tools.
Example Answers
I primarily use gdb for debugging C programs, as it allows me to set breakpoints and inspect variables. I also compile with -g for debug symbols and sometimes use Valgrind to detect memory leaks.
What strategies do you use for error handling in C, especially with pointers and memory allocation?
How to Answer
- 1
Always check the return value of memory allocation functions like malloc and calloc.
- 2
Set pointers to NULL after freeing them to avoid dangling pointers.
- 3
Implement error handling routines that log errors and possibly recover from them.
- 4
Use assertions to catch programming errors early during development.
- 5
Consider using smart pointers or wrapper functions in C++ for safer memory management.
Example Answers
I always check the return value of malloc to ensure that memory has been allocated successfully. If it's NULL, I handle the error gracefully, often by logging the issue and exiting the function with an error code.
Don't Just Read C Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your C Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What are pointers in C, and how do you use them? Can you provide an example where using pointers is advantageous?
How to Answer
- 1
Define what pointers are and their purpose in C programming.
- 2
Explain how to declare and initialize pointers clearly.
- 3
Discuss common operations involving pointers, such as dereferencing and pointer arithmetic.
- 4
Provide a specific example where pointers save memory or increase efficiency.
- 5
Conclude with the advantages of using pointers over regular variables.
Example Answers
Pointers in C are variables that store the memory address of another variable. They are declared using the '*' operator. For example, 'int *ptr;' declares a pointer to an int. Using pointers can be efficient in functions to avoid copying large data structures. For instance, passing a huge array by reference using pointers prevents overhead of copying the entire array.
Explain how memory management works in C. What are the common pitfalls associated with manual memory management?
How to Answer
- 1
Start by explaining how memory allocation and deallocation works in C using malloc and free.
- 2
Mention the distinction between stack and heap memory concepts.
- 3
Discuss common issues such as memory leaks, double freeing, and buffer overflows.
- 4
Highlight the importance of initializing pointers before use.
- 5
Conclude by emphasizing the need for careful tracking of allocated memory.
Example Answers
Memory management in C involves using functions like malloc to allocate memory and free to release it. Common pitfalls include forgetting to free memory, which leads to leaks, or freeing the same memory twice, causing crashes. Always initialize pointers to avoid undefined behavior and track allocations carefully.
How would you implement a linked list in C? What are the pros and cons of using a linked list?
How to Answer
- 1
Start by defining the structure for a linked list node.
- 2
Explain the basic operations: insert, delete, and traverse.
- 3
Discuss memory allocation for nodes using malloc.
- 4
Mention the advantages of dynamic size and ease of insertion/deletion.
- 5
List disadvantages like memory overhead and poor cache locality.
Example Answers
To implement a linked list in C, I would define a struct for the nodes that includes the data and a pointer to the next node. For operations, I would implement functions to insert a node at the head, delete a node, and traverse the list. The main advantage is dynamic sizing, while a downside is that each node requires extra memory for the pointer.
Can you walk me through how you would implement a binary search algorithm in C?
How to Answer
- 1
Start by explaining the concept of binary search and its requirements.
- 2
Mention that the input array must be sorted for the algorithm to work.
- 3
Outline the binary search steps: define pointers for the start and end of the array.
- 4
Describe the loop that continues until the start pointer exceeds the end pointer.
- 5
Explain how to calculate the middle index and compare the target value to the middle element.
Example Answers
Binary search is an efficient algorithm for finding a target in a sorted array. I would start by defining two pointers, one for the start and one for the end of the array. I would loop until the start pointer is greater than the end pointer, calculating the middle index. If the middle element is the target, I return the index. If the target is smaller, I move the end pointer to middle - 1, or if larger, I adjust the start pointer to middle + 1.
Explain the process of compiling a C program. What are preprocessor directives and how do they work?
How to Answer
- 1
Start by outlining the basic steps of compilation: preprocessing, compiling, assembling, and linking.
- 2
Define preprocessor directives and give examples such as #include and #define.
- 3
Explain the purpose of preprocessing, such as including header files and macro definitions.
- 4
Mention that preprocessor directives are handled before actual compilation starts.
- 5
Conclude by highlighting how preprocessor directives can affect the compiled code.
Example Answers
Compiling a C program involves several steps: first, the preprocessor handles directives like #include and #define, which prepare the code. Next, the compiler converts the source code into machine code. After that, the assembler translates it into object code, and finally, the linker combines all object files into an executable.
How do you create and manage threads in C? Can you give an example where multithreading improved performance?
How to Answer
- 1
Explain how to create threads using pthreads in C.
- 2
Discuss the use of pthread_create, pthread_join, and pthread_exit.
- 3
Mention thread synchronization techniques like mutexes and condition variables.
- 4
Provide a specific example relevant to multithreading benefits.
- 5
Keep your explanation concise and focused on performance improvements.
Example Answers
In C, I create and manage threads using the pthreads library. I use pthread_create to start a new thread and pthread_join to wait for it to finish. A good example is a program that processes multiple files simultaneously. By creating a separate thread for each file, I drastically reduce the total processing time, since they can be handled in parallel.
How would you implement a simple TCP server in C? What challenges might you encounter?
How to Answer
- 1
Start with socket creation using socket function
- 2
Use bind to associate the socket with an address
- 3
Call listen to prepare the socket for incoming connections
- 4
Use accept to handle incoming client connections
- 5
Discuss potential issues like blocking calls and error handling
Example Answers
To implement a simple TCP server in C, I'd create a socket with socket(), bind it to an address using bind(), listen for connections with listen(), and accept clients with accept(). A challenge might be managing multiple clients or handling errors gracefully.
What techniques do you use to optimize C code for performance?
How to Answer
- 1
Profile your code to identify bottlenecks before optimizing.
- 2
Use efficient algorithms and data structures suitable for your problem.
- 3
Minimize memory allocations and prefer stack memory over heap memory.
- 4
Leverage compiler optimizations and appropriate flags for speed.
- 5
Implement inline functions and avoid function call overhead where feasible.
Example Answers
I start by profiling the code to find performance bottlenecks. Based on that, I optimize algorithms and data structures for efficiency. I also minimize dynamic memory allocations and use stack memory when possible.
Don't Just Read C Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your C Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What are some common security vulnerabilities in C programming, and how can you prevent them?
How to Answer
- 1
Identify buffer overflows and discuss their risks
- 2
Mention null pointer dereferencing and its implications
- 3
Highlight the importance of input validation to prevent injection attacks
- 4
Emphasize proper memory management to avoid memory leaks and corruption
- 5
Advocate for using safer functions and libraries instead of unsafe ones
Example Answers
Common security vulnerabilities in C include buffer overflows and null pointer dereferencing. To prevent them, I ensure proper bounds checking and utilize safer functions like snprintf instead of sprintf. Additionally, I validate all input to prevent injections and manage memory carefully to avoid leaks.
What is a C standard library function that you frequently use, and why is it useful?
How to Answer
- 1
Choose a specific function you use often.
- 2
Explain its purpose and give a practical example.
- 3
Highlight its advantages in terms of efficiency or convenience.
- 4
Relate it to a real project where you implemented it.
- 5
Keep your answer concise and focused.
Example Answers
I frequently use the `malloc` function because it allows dynamic memory allocation. In one project, I needed to handle varying amounts of data, and `malloc` enabled me to allocate memory on the heap, ensuring that I used only what I needed.
How do you handle file input and output in C, and what are some common challenges you've encountered?
How to Answer
- 1
Explain the use of fopen, fread, fwrite, fclose for file operations.
- 2
Mention error handling using errno and feof.
- 3
Discuss common issues like file permissions and buffering.
- 4
Provide an example of a specific challenge you've faced, such as reading large files.
- 5
Keep your explanation concise and structured.
Example Answers
I handle file I/O in C primarily using the standard library functions. I use fopen to open a file, and then I use fread or fwrite for reading and writing data. It's important to check for errors by verifying the return values and using errno. A common challenge I've faced is managing the right file permissions, which can prevent my program from accessing a file as expected. I ensure to always close files with fclose to avoid memory leaks.
Behavioral Interview Questions
Can you describe a situation where you had to work on a C project with a team? What was your role and how did you collaborate with others?
How to Answer
- 1
Identify a specific C project you worked on with a team
- 2
Clearly state your role and responsibilities
- 3
Highlight how you communicated with team members, such as using version control or meetings
- 4
Emphasize any challenges you faced and how you resolved them as a group
- 5
Mention the outcome of the project and what you learned from the experience
Example Answers
In a recent project, I worked on developing a firmware application for a microcontroller in C. I was responsible for the communication module. We used Git for version control and had regular stand-ups to discuss progress. We faced a challenge with data synchronization, which we solved by implementing a threaded approach, resulting in a successful product launch.
Tell me about a particularly challenging problem you faced while developing in C. How did you approach it and what was the outcome?
How to Answer
- 1
Identify a specific technical challenge you encountered.
- 2
Describe the context briefly, focusing on why it was difficult.
- 3
Explain your problem-solving process step by step.
- 4
Highlight any tools or methods you used to resolve the issue.
- 5
Conclude with the outcome and any lessons learned from the experience.
Example Answers
I faced a memory leak issue in a network application. I used Valgrind to analyze memory usage, which revealed that I was not freeing allocated memory in several functions. I systematically went through the code, added the necessary free() calls, and reran Valgrind, which showed no leaks. This improved the application's stability and performance significantly.
Don't Just Read C Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your C Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Can you give an example of a conflict or disagreement you had with a colleague during a project? How did you handle it and what was the result?
How to Answer
- 1
Select a specific situation that clearly illustrates the conflict.
- 2
Explain your colleague's perspective and your own without assigning blame.
- 3
Highlight the steps you took to resolve the disagreement, focusing on communication.
- 4
Discuss the outcome positively, showing what you learned or how it improved the project.
- 5
Keep it professional and avoid mentioning personal conflicts.
Example Answers
During a project, my colleague and I disagreed on the implementation of a data structure. They preferred dynamic memory for flexibility, while I advocated for static allocation for performance. I listened to their reasoning, then explained my perspective. We decided to test both approaches in a small prototype. Ultimately, we chose to implement my static method since it was more efficient for our use case, leading to a successful and timely project completion.
Describe a time when you had to meet a tight deadline while working on a C project. How did you prioritize your tasks?
How to Answer
- 1
Identify a specific project with a clear deadline.
- 2
Explain your planning process and how you determined priority tasks.
- 3
Mention any tools or techniques you used for task management.
- 4
Discuss how you communicated with your team during this period.
- 5
Reflect on the outcome and what you learned from the experience.
Example Answers
In a recent project, I had to implement a new feature in C within a week. I first listed the critical tasks needed to complete the feature, focusing on the most impactful changes. I used a simple Kanban board to keep track of progress. I communicated daily with my team to ensure alignment and address any blockers. We delivered the feature on time and it performed well in testing.
Give an example of a time when you had to adapt to significant changes during a C project. How did you manage it?
How to Answer
- 1
Identify a specific project where changes occurred.
- 2
Explain the nature of the changes and their impact.
- 3
Describe your immediate response to the changes.
- 4
Discuss how you communicated with your team or stakeholders.
- 5
Highlight the outcome and any lessons learned.
Example Answers
In a project developing a network application in C, the requirements shifted from a simple TCP protocol to supporting both TCP and UDP. I quickly researched the differences and proposed a design that could handle both. I communicated the new architecture to the team and we held a meeting to align on adjustments. As a result, we met the new deadline and delivered a robust application.
Describe an instance where you took the initiative to improve a C project or process.
How to Answer
- 1
Identify a specific project or process you improved.
- 2
Explain the problem you noticed and why it was important.
- 3
Discuss the action you took and how you implemented it.
- 4
Highlight the outcome and improvements resulting from your initiative.
- 5
Keep it concise and focus on your direct contribution.
Example Answers
In a project for embedded systems, I noticed our memory usage was inefficient. I took the initiative to refactor critical code sections, optimizing our data structures, which reduced memory usage by 30%. This not only improved performance but also allowed us to add new features.
Have you ever led a project involving multiple team members? How did you ensure that the project stayed on track?
How to Answer
- 1
Briefly describe the project and your role in leading it
- 2
Mention specific tools or methods used for communication and tracking progress
- 3
Highlight how you assigned tasks and monitored deadlines
- 4
Discuss any challenges faced and how you addressed them
- 5
Conclude with the positive outcome or lessons learned from the experience
Example Answers
I led a team of four in developing a C application for data processing. We used Jira for tracking tasks and held weekly stand-ups to discuss progress. I assigned tasks based on each member’s strengths and ensured deadlines were met by setting clear milestones. When we encountered a significant bug, I organized an extra session to address it quickly, which helped us stay on schedule. The project was completed successfully and on time.
Situational Interview Questions
Imagine you are given a large C codebase with a critical bug affecting performance. How would you go about diagnosing and fixing the issue?
How to Answer
- 1
Identify the performance bottleneck using profiling tools like gprof or Valgrind.
- 2
Review recent code changes that might have introduced the bug.
- 3
Check for inefficient algorithms or data structures being used.
- 4
Isolate the problematic code by creating minimal test cases.
- 5
Make incremental changes and measure performance improvements.
Example Answers
I would start by profiling the application to find the slowest functions. After identifying the bottleneck, I would check the recent commits and assess if there are any relevant changes. If needed, I would rewrite inefficient sections using better algorithms and continually test the performance until the issue is resolved.
Suppose you need to add a major feature to an existing C application. How would you design and implement the feature while ensuring minimal disruption?
How to Answer
- 1
Review existing architecture to understand dependencies and integration points
- 2
Use feature flags to control feature rollout and avoid breaking existing functionality
- 3
Implement comprehensive unit tests for new feature to ensure stability
- 4
Conduct code reviews and involve team members for feedback on the design
- 5
Document changes thoroughly to aid future maintenance and onboarding
Example Answers
First, I would analyze the existing codebase to identify where the new feature would fit, ensuring I understand existing dependencies. I would use feature flags to introduce the feature gradually, and create detailed unit tests to verify that everything works as expected without disrupting existing functionality.
Don't Just Read C Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your C Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
You're asked to review a colleague's C code that follows different stylistic conventions. How would you address your feedback?
How to Answer
- 1
Start with positive feedback on what works well in the code.
- 2
Identify specific stylistic issues clearly and provide examples.
- 3
Suggest alternative conventions or best practices with rationale.
- 4
Encourage discussion to understand their choices and perspectives.
- 5
Offer to assist with revisions or collaborative improvement.
Example Answers
I would begin by highlighting the good parts of the code, like its logic and functionality. Then, I would point out specific stylistic inconsistencies, such as variable naming or indentation. I would recommend adhering to a style guide like K&R or MISRA and explain why it's important for consistency. I would also invite my colleague to share their views on their stylistic choices and offer to help them revise the code together.
You are tasked with refactoring a C module that hasn’t been updated in years. What steps would you take to ensure modern coding standards are met?
How to Answer
- 1
Review current code for outdated practices and syntax.
- 2
Run static analysis tools to identify potential issues.
- 3
Update to use C11 or newer features where applicable.
- 4
Implement unit tests to ensure functionality is preserved.
- 5
Document changes and update coding standards documentation.
Example Answers
First, I would analyze the current codebase to spot outdated syntax and practices. Then, I would use static analysis tools like Splint to highlight bugs or security vulnerabilities. After that, I would refactor the code to incorporate features from C11, ensuring modern coding standards. I would write unit tests to confirm that the module's functionality remains intact, and finally, I would document all changes made to the coding standards.
Your C application is running slower than expected on large datasets. How would you identify and implement performance improvements?
How to Answer
- 1
Profile the application to find bottlenecks using tools like gprof or Valgrind.
- 2
Review algorithms and data structures for inefficiencies; consider alternative approaches.
- 3
Optimize memory usage; reduce allocations and free unnecessary memory.
- 4
Consider parallelism; use multithreading or vectorization where applicable.
- 5
Compile with optimization flags to improve performance (e.g., -O2, -O3).
Example Answers
First, I would use gprof to profile the application and identify where the most time is spent. Then, I would analyze the algorithms in use and see if switching to a more efficient algorithm could help. Additionally, I would review the memory usage and minimize allocations where possible. Finally, I would compile the application with -O3 for better optimization.
A team member insists on implementing a C feature a certain way, which you believe is inefficient. How would you address this situation?
How to Answer
- 1
Listen to their reasoning before responding
- 2
Gather data to support your perspective
- 3
Suggest a collaborative approach to evaluate both solutions
- 4
Be respectful and open-minded in your discussion
- 5
Propose a small prototype to test both methods
Example Answers
I would first listen to my team member's reasoning for their approach. After that, I'd point out the specific inefficiencies and share data from similar past experiences to support my view. I would suggest that we collaborate on a simple prototype to see which implementation performs better.
You need to develop a C application within strict hardware resource limits. How would you approach this challenge?
How to Answer
- 1
Understand the specific resource limits such as memory and CPU usage
- 2
Optimize data structures and algorithms for efficiency
- 3
Use fixed-size buffers and avoid dynamic memory allocation
- 4
Profile the application to identify resource bottlenecks
- 5
Consider using lower-level programming techniques for critical sections
Example Answers
I start by clearly defining the resource constraints. I make sure to optimize my algorithms and choose data structures that minimize memory usage. I also prefer fixed-size arrays over dynamic memory allocation to avoid fragmentation.
You must choose between two C libraries for implementing a critical feature. What factors would you consider in making your decision?
How to Answer
- 1
Evaluate performance benchmarks of both libraries for the specific use case.
- 2
Consider the library's documentation and support community for troubleshooting.
- 3
Check for licensing compatibility with your project requirements.
- 4
Assess the maintenance status and update frequency of each library.
- 5
Review any existing integrations or dependencies within your current codebase.
Example Answers
I would first look at the performance benchmarks specific to my use case to determine which library performs better. Then, I would consider the quality of the documentation and community support to ensure any issues can be resolved quickly.
You've noticed that an old C codebase lacks proper testing. How would you introduce unit tests effectively?
How to Answer
- 1
Assess the existing codebase to identify key modules and functions that require testing
- 2
Start with the most critical parts of the code that are frequently used or have complex logic
- 3
Use a testing framework suitable for C, like CUnit or Unity, to write automated tests
- 4
Gradually add tests to the codebase, ensuring each new feature or bug fix includes tests
- 5
Document the testing process and encourage the team to adopt testing as a standard practice.
Example Answers
I would first review the codebase to find the most critical parts that need tests. Then, I would choose a testing framework like CUnit to create unit tests for those components, prioritizing frequently used or complex functions.
C Developer Position Details
Recommended Job Boards
ZipRecruiter
www.ziprecruiter.com/Jobs/C-DeveloperThese job boards are ranked by relevance for this position.
Related Positions
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates