Top 30 Compiler Interview Questions and Answers [Updated 2025]
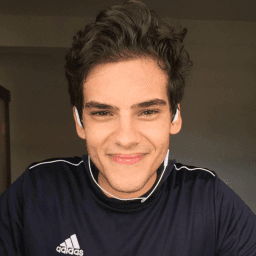
Andre Mendes
•
March 30, 2025
Preparing for a compiler role interview can be daunting, but we’ve got you covered with a comprehensive list of the most common questions you might face. In this updated 2025 guide, you'll find not only the key questions but also example answers and insightful tips on how to respond effectively. Dive in to boost your confidence and ace that interview with ease!
Download Compiler Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Compilerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Compiler Interview Questions
Situational Interview Questions
Imagine you need to improve the runtime performance of a compiled program. What strategies would you consider?
How to Answer
- 1
Analyze the performance bottlenecks using profiling tools
- 2
Consider optimizing algorithms for better efficiency
- 3
Explore compiler optimizations like loop unrolling or inlining
- 4
Assess memory usage and reduce unnecessary allocations
- 5
Parallelize tasks where possible to leverage multi-core processors
Example Answers
First, I would profile the application to identify where most time is spent. Then, I would optimize the algorithms used in those hotspots, perhaps replacing a quadratic algorithm with a linear one if possible.
You find a performance issue during code generation. How would you approach debugging it?
How to Answer
- 1
Profile the code generation to identify slow parts.
- 2
Check for common inefficiencies in the generated code.
- 3
Examine data structures and algorithms used in code generation.
- 4
Consider compiler optimization flags and options.
- 5
Use debugging tools or logging to gather more data.
Example Answers
First, I would use a profiler to find bottlenecks in the code generation process. Then, I'd analyze the generated output for inefficiencies, focusing on loops and memory usage. If necessary, I'd explore different optimization flags to see if they improve performance.
Good Candidates Answer Questions. Great Ones Win Offers.
Reading sample answers isn't enough. Top candidates practice speaking with confidence and clarity. Get real feedback, improve faster, and walk into your next interview ready to stand out.
Master your interview answers under pressure
Boost your confidence with real-time practice
Speak clearly and impress hiring managers
Get hired faster with focused preparation
Used by thousands of successful candidates
You have a tight deadline to implement a new language feature in the compiler. How would you prioritize your tasks?
How to Answer
- 1
Identify the core functionality needed for the feature.
- 2
Break down the tasks into smaller, manageable parts.
- 3
Assess dependencies between tasks and tackle critical ones first.
- 4
Communicate with the team to align on priorities and expectations.
- 5
Focus on delivering a working prototype before refining details.
Example Answers
First, I would identify the essential functionality of the new feature and break it down into smaller tasks. Then, I would prioritize tasks based on dependencies, ensuring that critical paths are handled first. Maintaining clear communication with the team would help keep everyone aligned on priorities, while ensuring I deliver a working prototype before polishing the feature further.
If a team member disagrees with your approach to a compiler design issue, how would you handle the situation?
How to Answer
- 1
Listen actively to their concerns without interrupting.
- 2
Ask clarifying questions to understand their perspective better.
- 3
Acknowledge valid points and show openness to different ideas.
- 4
Discuss the trade-offs and benefits of both approaches collaboratively.
- 5
Aim for a consensus or propose a compromise to move forward.
Example Answers
I would first listen to my colleague's concerns, ensuring I fully understand their position. Then, I'd ask questions to clarify their points and see if there are valid aspects to consider. After discussing the pros and cons together, I would look for a compromise that blends our ideas effectively.
Suppose you encounter an undefined behavior in a compiled program. What steps would you take to address it?
How to Answer
- 1
Identify the location of the undefined behavior using debugging tools.
- 2
Check the compiler documentation for potential causes of undefined behavior.
- 3
Isolate the problematic code and create a minimal reproducible example.
- 4
Use sanitizers or static analysis tools to detect the issue.
- 5
Refactor the code to eliminate the undefined behavior or rewrite it to ensure defined behavior.
Example Answers
First, I would use a debugger to pinpoint where the undefined behavior occurs. Then I'd refer to the compiler's documentation to understand what could lead to this issue. After that, I'd isolate the code and create a minimal example to better analyze the problem. Using a sanitizer helps catch these issues, and finally, I would refactor the code accordingly.
How would you approach a code review for a fellow programmer's implementation of a new optimization pass?
How to Answer
- 1
Understand the goals of the optimization pass clearly and its intended impact on performance.
- 2
Check the logic of the implementation against the algorithm it is supposed to optimize.
- 3
Look for adherence to code style guides and best practices in coding style.
- 4
Evaluate test coverage thoroughly for correctness and edge cases in the optimization.
- 5
Provide constructive feedback focusing on both strengths and areas for improvement.
Example Answers
I would first review the purpose of the optimization pass to ensure it aligns with performance goals. Then I would analyze the implementation logic to verify correctness against the expected algorithm. I'd also check coding standards and assess test coverage to ensure edge cases are handled effectively.
If you were assigned a legacy codebase for a compiler with little documentation, how would you proceed?
How to Answer
- 1
Start by building an understanding of the code structure and dependencies.
- 2
Run existing tests to see what the codebase currently does and identify any immediate issues.
- 3
Use tools like call graphs or static analysis to explore relationships in the code.
- 4
Document findings and assumptions as you go to build your own reference.
- 5
Engage with any available developers or users to gather insights about the codebase.
Example Answers
I would begin by exploring the code structure and running existing tests to gauge functionality. Next, I’d use static analysis tools to understand the relationships within the code. I would keep notes on what I find and reach out to any former developers for insights.
You need to choose between a faster but more complex algorithm versus a simpler but slower one for a compiler feature. What factors would influence your decision?
How to Answer
- 1
Consider the trade-off between development time and performance impact on the compiler.
- 2
Evaluate the complexity of implementation and maintenance of the algorithm.
- 3
Assess the target audience and usage patterns of the compiler feature.
- 4
Think about the scalability and long-term implications of the algorithm choice.
- 5
Analyze performance benchmarks and potential bottlenecks based on algorithm choice.
Example Answers
I would consider the implementation complexity versus expected performance gains. If the faster algorithm significantly improves performance in critical areas without overwhelming maintenance costs, I would choose it.
How would you incorporate user feedback into further iterations of a compiler you are developing?
How to Answer
- 1
Gather feedback through surveys and user interviews at regular intervals.
- 2
Prioritize feedback based on impact and frequency of similar requests.
- 3
Implement changes in small iterations to test their effectiveness before wider rollout.
- 4
Maintain open communication with users about what changes are being made and why.
- 5
Use analytics and performance metrics to evaluate the effect of changes based on user feedback.
Example Answers
I would regularly conduct user surveys to collect feedback on the compiler's usability and features. This feedback would be categorized and prioritized, allowing us to tackle the most impactful changes first. I would implement these changes incrementally to assess their impact on user experience.
Behavioral Interview Questions
Describe a time you worked with a team to resolve a challenging issue in the compiler development process.
How to Answer
- 1
Identify a specific challenge your team faced.
- 2
Explain the collaborative approach taken to address the issue.
- 3
Highlight your role and contributions in the team effort.
- 4
Discuss the outcome and what was learned from the experience.
- 5
Keep the focus on teamwork and problem-solving techniques.
Example Answers
In my last project, we faced a significant performance regression in our compiler's optimization phase. Our team held brainstorming sessions to identify the root cause. I suggested profiling the optimization passes and led a subgroup to analyze the results. Together, we discovered a misconfigured pass that was slowing down execution. After applying the fix, our compiler's performance improved by 20%. This collaboration taught me the importance of thorough profiling and communication.
Can you provide an example of a complex bug you encountered in a compiler? How did you approach resolving it?
How to Answer
- 1
Clearly describe the bug and its impact on the compiler's output
- 2
Explain the process of identifying the source of the bug
- 3
Discuss the tools or methods you used to investigate
- 4
Outline the steps taken to fix the bug
- 5
Reflect on what you learned from the experience
Example Answers
In my last project, I encountered a bug where the optimizer incorrectly altered a loop, causing incorrect results. I noticed that the output for specific input cases changed unexpectedly. I used a combination of step-through debugging and added logging to identify that the transformation was mishandling loop invariants. After pinpointing the faulty transformation rule in the optimizer, I corrected the logic and added test cases to cover those scenarios. This experience taught me the importance of validating assumptions made during optimizations.
Good Candidates Answer Questions. Great Ones Win Offers.
Reading sample answers isn't enough. Top candidates practice speaking with confidence and clarity. Get real feedback, improve faster, and walk into your next interview ready to stand out.
Master your interview answers under pressure
Boost your confidence with real-time practice
Speak clearly and impress hiring managers
Get hired faster with focused preparation
Used by thousands of successful candidates
How do you handle changes in project requirements when working on compiler optimization?
How to Answer
- 1
Stay flexible and adaptive to new requirements as they arise
- 2
Prioritize changes by assessing their impact on performance and timelines
- 3
Communicate clearly with your team about changes and expected outcomes
- 4
Use version control and feature branching to manage changes effectively
- 5
Document all changes in requirements and their implications for future reference
Example Answers
I remain flexible and adapt my optimization strategies as new requirements come in. I assess their impact on performance and work with the team to prioritize the most critical changes. This way, we can maintain our timeline and ensure we deliver effective optimizations.
Tell me about a time you led a project or a team related to compiler design.
How to Answer
- 1
Describe the project clearly and its objectives.
- 2
Focus on your leadership role and specific actions you took.
- 3
Mention any challenges you faced and how you overcame them.
- 4
Highlight the outcome of the project and its impact.
- 5
Include any collaborative efforts or skills utilized in the process.
Example Answers
In my final year at university, I led a team developing a mini-compiler for a custom scripting language. My role involved coordinating the development schedule and ensuring everyone understood their tasks. We faced significant challenges with optimizations, but I organized brainstorming sessions which helped us find solutions. Ultimately, we delivered a functional compiler ahead of schedule, and it received positive feedback from our professors.
Describe a situation where you had to explain a technical compiler concept to a non-technical audience.
How to Answer
- 1
Start with a relatable analogy to make the concept easier to understand.
- 2
Use simple language, avoiding jargon and technical terms.
- 3
Focus on the practical implications of the concept, not the technical details.
- 4
Encourage questions to clarify any confusion.
- 5
Summarize the main point at the end to reinforce understanding.
Example Answers
I once explained what a compiler does by comparing it to a translator at an airport. Just like how a translator helps a traveler communicate with locals, a compiler translates high-level code into machine code that the computer can understand. I emphasized that this process is crucial for software to run smoothly.
Have you ever taken the initiative to learn a new technology that benefited your work on compilers? Tell me about it.
How to Answer
- 1
Identify a specific technology you learned.
- 2
Explain why you chose to learn it and its relevance to compilers.
- 3
Describe how you applied this technology to a project.
- 4
Share any measurable outcomes or improvements from your learning.
- 5
Keep the response focused on your initiative and the positive impact.
Example Answers
I learned LLVM to improve our compiler's optimization passes. I recognized LLVM's capabilities for advanced optimizations, so I took a course. I integrated LLVM into our project, which resulted in a 30% increase in compile time efficiency.
Can you describe a time when you had to learn a new programming language quickly for a compiler project?
How to Answer
- 1
Identify a specific project where you needed to learn quickly.
- 2
Explain the context and urgency of learning the language.
- 3
Discuss the resources or strategies you used to learn.
- 4
Share how you applied your new knowledge in the project.
- 5
Reflect on the outcome and what you learned from the experience.
Example Answers
During my internship at XYZ Corp, I had to learn Rust for a compiler optimization project. I had just one week to become proficient, so I focused on online tutorials and the Rust documentation. I applied what I learned by contributing to the codebase, and we improved the compiler's performance by 20%. This experience taught me how to adapt quickly and leverage community resources effectively.
Technical Interview Questions
What are the different parsing techniques you are familiar with and when would you use each?
How to Answer
- 1
List common parsing techniques such as top-down and bottom-up parsing.
- 2
Explain the differences between techniques like LL, LR, and recursive descent.
- 3
Discuss the advantages and disadvantages of each method.
- 4
Link the choice of parsing technique to the type of language being parsed.
- 5
Use specific examples from your experience or studies.
Example Answers
I am familiar with several parsing techniques: LL parsing is top-down and works well for deterministic contexts, but it struggles with certain ambiguous grammars. LR parsing is bottom-up and can handle a wider range of grammars, making it suitable for complex languages like C or C++. I would use LL for simpler, clear grammars and LR for more complex use cases.
Can you explain the differences between loop unrolling and loop fusion in compiler optimization?
How to Answer
- 1
Define both loop unrolling and loop fusion clearly.
- 2
Explain the goals of each optimization technique.
- 3
Discuss the impact on performance for both methods.
- 4
Provide a brief example for each technique.
- 5
Highlight situations where one might be preferred over the other.
Example Answers
Loop unrolling is a technique that reduces the overhead of loop control by increasing the number of operations inside the loop. In contrast, loop fusion combines two adjacent loops that iterate over the same data to reduce overhead and improve cache performance. The goal of unrolling is to minimize the loop overhead, while fusion aims to enhance locality. For instance, unrolling a loop that sums an array can cut down the number of iterations and checks. Fusion is applicable when two loops have similar iteration counts and share variables.
Good Candidates Answer Questions. Great Ones Win Offers.
Reading sample answers isn't enough. Top candidates practice speaking with confidence and clarity. Get real feedback, improve faster, and walk into your next interview ready to stand out.
Master your interview answers under pressure
Boost your confidence with real-time practice
Speak clearly and impress hiring managers
Get hired faster with focused preparation
Used by thousands of successful candidates
What are context-free grammars and how do they relate to compiler design?
How to Answer
- 1
Define context-free grammar (CFG) clearly
- 2
Explain how CFGs are used to define the syntax of programming languages
- 3
Discuss the role of parsers that use CFGs in compilers
- 4
Highlight the importance of CFGs in syntax analysis phase
- 5
Mention how CFGs can help in creating abstract syntax trees (ASTs)
Example Answers
Context-free grammars are formal rules that describe the syntax of programming languages. They define how strings of symbols can be generated and are crucial for the parsing phase in compilers, which analyzes code to build structures like parse trees or abstract syntax trees.
Describe the process of code generation in a compiler. What are the key steps involved?
How to Answer
- 1
Begin by mentioning the context of code generation in the compilation process.
- 2
Outline the primary inputs for code generation, like the intermediate representation.
- 3
List the key steps including instruction selection, register allocation, and instruction scheduling.
- 4
Emphasize the importance of optimization during code generation.
- 5
Conclude with the output of the code generation phase, typically machine code or assembly.
Example Answers
Code generation is the final phase of a compiler where the intermediate representation is translated to machine code. The key steps include selecting appropriate machine instructions, allocating registers for variables, scheduling instructions for optimal performance, and applying any necessary code optimizations. The result is executable machine code suitable for the target architecture.
How does a compiler handle semantic errors? Can you give an example?
How to Answer
- 1
Define what semantic errors are clearly.
- 2
Explain how the compiler detects these errors during compilation.
- 3
Mention how the compiler reports these errors back to the programmer.
- 4
Give a specific example of a semantic error, like type mismatches.
- 5
Conclude with the importance of semantic checks in ensuring program correctness.
Example Answers
Semantic errors occur when the program is syntactically correct but has logical inconsistencies. The compiler detects these during the semantic analysis phase, where it checks type compatibility. For example, trying to add an integer to a string would result in a semantic error, and the compiler will report this to the programmer.
What data structures do you find most useful in the implementation of compilers?
How to Answer
- 1
Identify key phases of the compiler where data structures play a role
- 2
Mention specific data structures used in parsing, semantic analysis, and code generation
- 3
Explain why each data structure is appropriate for its use case
- 4
Use examples from actual compilers if possible
- 5
Keep your answer focused and avoid unrelated data structures
Example Answers
In the parsing phase, I find that trees, particularly abstract syntax trees (ASTs), are vital for representing the hierarchical structure of source code. They allow for efficient traversals and modifications during compilation.
What compiler toolchains are you familiar with, and how have you used them in your projects?
How to Answer
- 1
List specific compiler toolchains you know like GCC, Clang, or MSVC.
- 2
Mention any programming languages you have compiled using these toolchains.
- 3
Describe a project where you utilized these toolchains.
- 4
Highlight any challenges you faced and how you overcame them.
- 5
Discuss any optimization techniques or flags you used during compilation.
Example Answers
I am familiar with GCC and Clang. I used GCC for a C project where I implemented a custom memory manager. I faced issues with standard compliance but solved them by using specific flags to enable GNU extensions.
What are the differences between static and dynamic type systems in programming languages?
How to Answer
- 1
Define static and dynamic type systems clearly.
- 2
Highlight key characteristics of each type system.
- 3
Provide examples of languages that utilize each type system.
- 4
Explain the implications on error detection and performance.
- 5
Mention the flexibility and safety trade-offs for developers.
Example Answers
Static type systems check variable types at compile time, like in Java or C++, enhancing safety and performance but reducing flexibility. In contrast, dynamic type systems, seen in Python and JavaScript, allow type checks at runtime, providing more flexibility but possibly leading to runtime errors.
What is an Intermediate Representation (IR) in compilers and why is it important?
How to Answer
- 1
Define what Intermediate Representation is in clear terms.
- 2
Explain the role of IR in the compilation process.
- 3
Discuss why IR enables optimizations and portability.
- 4
Mention examples of common IRs in existing compilers.
- 5
Keep the explanation concise and focused on the main points.
Example Answers
An Intermediate Representation (IR) is a data structure used in compilers to represent source code during the compilation process. It is important because it acts as a bridge between the source code and the target machine code, allowing for optimizations and making the compiler more modular.
What is LLVM and how does it facilitate compiler construction?
How to Answer
- 1
Define LLVM clearly as a collection of modular and reusable compiler and toolchain technologies.
- 2
Explain its main components such as the LLVM intermediate representation (IR) and the optimization passes.
- 3
Highlight how LLVM simplifies backend development by providing a standard interface and numerous tools.
- 4
Mention cross-platform support and the ability to target multiple architectures.
- 5
Conclude with how it allows for easier experimentation with new language features and optimizations.
Example Answers
LLVM stands for Low-Level Virtual Machine and it is a set of modular compiler and toolchain technologies. It uses an intermediate representation (IR) that is both platform-independent and allows for advanced optimizations throughout the compilation process. This modularity lets developers focus on building frontends for new languages without worrying about the backend specifics.
Good Candidates Answer Questions. Great Ones Win Offers.
Reading sample answers isn't enough. Top candidates practice speaking with confidence and clarity. Get real feedback, improve faster, and walk into your next interview ready to stand out.
Master your interview answers under pressure
Boost your confidence with real-time practice
Speak clearly and impress hiring managers
Get hired faster with focused preparation
Used by thousands of successful candidates
How would you implement static analysis features in a compiler?
How to Answer
- 1
Define the specific static analysis checks you want to implement.
- 2
Integrate analysis checks at appropriate stages in the compilation process.
- 3
Use an Abstract Syntax Tree (AST) for easier code analysis.
- 4
Provide informative warnings or errors to help developers fix issues.
- 5
Consider performance implications and ensure analysis does not slow down compilation significantly.
Example Answers
I would start by defining key static analysis checks like unused variable detection and null reference checks. Then, I'd integrate these checks during the semantic analysis phase, leveraging the AST to traverse the code and identify issues. I'd ensure that any warnings are clear and actionable, helping the developer to understand the potential problems.
What tools do you typically use for debugging compiler-related issues?
How to Answer
- 1
Mention specific debugging tools you've used.
- 2
Explain how these tools help in identifying compiler issues.
- 3
Include any integrated tools within IDEs that assist in debugging.
- 4
Share experiences where a tool significantly helped solve a problem.
- 5
Be prepared to discuss the limitations of certain tools.
Example Answers
I typically use GDB for debugging compiler-related issues. It allows me to step through code and examine variable states, which is crucial for understanding where a compiler might be producing incorrect outputs.
Compiler Position Details
Recommended Job Boards
CareerBuilder
www.careerbuilder.com/jobs/compiler-engineerZipRecruiter
www.ziprecruiter.com/Jobs/Compiler-EngineerThese job boards are ranked by relevance for this position.
Related Positions
Similar positions you might be interested in.
Good Candidates Answer Questions. Great Ones Win Offers.
Master your interview answers under pressure
Boost your confidence with real-time practice
Speak clearly and impress hiring managers
Get hired faster with focused preparation
Used by thousands of successful candidates
Good Candidates Answer Questions. Great Ones Win Offers.
Master your interview answers under pressure
Boost your confidence with real-time practice
Speak clearly and impress hiring managers
Get hired faster with focused preparation
Used by thousands of successful candidates