Top 30 Front End Developer Interview Questions and Answers [Updated 2025]
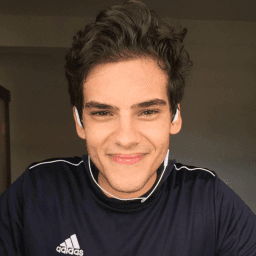
Andre Mendes
•
March 30, 2025
Preparing for a front end developer interview can be daunting, but we're here to help you ace it. In this blog post, we've compiled the most common interview questions for the Front End Developer role, complete with example answers and practical tips for crafting your responses. Boost your confidence and get ready to impress with insights that will set you apart from the competition.
Get Front End Developer Interview Questions PDF
Get instant access to all these Front End Developer interview questions and expert answers in a convenient PDF format. Perfect for offline study and interview preparation.
Enter your email below to receive the PDF instantly: