Top 30 Gopher Interview Questions and Answers [Updated 2025]
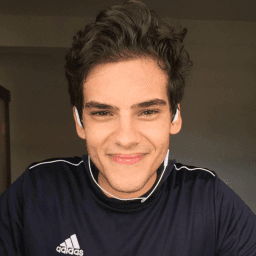
Andre Mendes
•
March 30, 2025
Preparing for a Gopher interview? Our latest blog post is your go-to resource for the most common questions encountered in this role. Dive into expertly crafted example answers and insightful tips to ensure you respond with confidence and clarity. Whether you're a seasoned professional or a newcomer, this guide will equip you with the strategies needed to impress your interviewers and secure that position.
Download Gopher Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Gopherinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Gopher Interview Questions
Technical Interview Questions
How do you write and run tests in Go? Can you give an example?
How to Answer
- 1
Use the testing package in Go to create tests.
- 2
Write your test functions starting with 'Test' followed by the function's name.
- 3
Use 't *testing.T' to report failures in the test.
- 4
Run tests using the command 'go test' in the terminal.
- 5
Keep tests concise and focused on specific behaviors.
Example Answers
In Go, I use the testing package to write my tests. I define a function like 'func TestMyFunction(t *testing.T)' where I can check the output using 't.Error()' if the result is incorrect. To run the tests, I simply execute 'go test'.
Explain how you would implement a basic HTTP server in Go.
How to Answer
- 1
Start by importing the net/http package.
- 2
Define a handler function that responds to HTTP requests.
- 3
Use http.HandleFunc to register the handler with a specific route.
- 4
Call http.ListenAndServe with the desired port and nil as the handler.
- 5
Keep the implementation simple and focus on core functionality.
Example Answers
First, I would import the net/http package. I would then create a handler function that writes a simple response. Next, I'd use http.HandleFunc to map a route like "/" to my handler. Finally, I'd call http.ListenAndServe on port 8080 to start the server.
Don't Just Read Gopher Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Gopher interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
How would you leverage Go's concurrency features to handle multiple tasks simultaneously?
How to Answer
- 1
Explain goroutines and how they allow for lightweight concurrent execution.
- 2
Discuss channels for safe communication between goroutines.
- 3
Mention the use of the 'sync' package for coordinating between goroutines.
- 4
Provide a simple example that demonstrates concurrent processing.
- 5
Highlight the benefits of concurrency in improving performance and responsiveness.
Example Answers
I would use goroutines to handle multiple tasks at once, as they allow creating thousands of concurrent tasks without significant memory overhead. For communication, I would use channels to pass data safely between them, ensuring no race conditions occur.
What are best practices for handling errors in Go?
How to Answer
- 1
Always check for errors immediately after calling a function that returns an error.
- 2
Use the error wrapping feature to provide context about the error.
- 3
Implement custom error types for complex error handling needs.
- 4
Log errors appropriately instead of panicking in production code.
- 5
Consider returning errors to the caller for better error propagation.
Example Answers
In Go, I always check for errors right after function calls that return them. For example, I might do `if err != nil { return err }` to propagate the error up.
How does Go handle dependencies, and how would you use Go modules?
How to Answer
- 1
Explain Go's dependency management system clearly
- 2
Mention the use of go.mod and go.sum files
- 3
Discuss how to add and update dependencies with commands
- 4
Highlight the importance of modules for versioning
- 5
Provide an example of initializing a module and getting a dependency
Example Answers
Go manages dependencies using modules, which are defined by a go.mod file. You can add a dependency using the 'go get' command, which automatically updates the go.mod and go.sum files. For example, to add the 'example.com/pkg' dependency, I would run 'go get example.com/pkg'.
What data structures are available in Go, and when would you use them?
How to Answer
- 1
Familiarize yourself with Go's built-in data structures: arrays, slices, maps, and structs.
- 2
Explain the use case for each data structure when responding.
- 3
Mention performance characteristics like memory usage, access time, and ease of use.
- 4
Be ready to discuss how to create and manipulate these structures in Go.
- 5
Consider using examples from projects or scenarios to illustrate your point.
Example Answers
Go provides several data structures, such as arrays for fixed-size collections, slices for dynamic arrays, maps for key-value pairs, and structs for complex data types. I would use slices when I need a resizable array and maps when I need to quickly access data via keys.
Explain how interfaces work in Go and provide an example of when you would use them.
How to Answer
- 1
Start with a clear definition of interfaces in Go.
- 2
Mention how interfaces allow for polymorphism.
- 3
Provide a simple example that illustrates interface use.
- 4
Discuss a specific scenario where interfaces simplify code.
- 5
Keep the explanation focused on key concepts without unnecessary jargon.
Example Answers
In Go, an interface is a type that defines a contract. Any type that implements the methods declared in the interface satisfies that interface. For example, if we have an interface 'Shape' with a method 'Area', both 'Circle' and 'Square' can implement 'Area', allowing us to treat them as 'Shape'. This is useful in a drawing application where you can create a slice of 'Shape' and iterate over it to calculate the total area.
What are goroutines in Go, and how do they differ from threads?
How to Answer
- 1
Define goroutines as lightweight, concurrent functions in Go.
- 2
Mention how they are managed by the Go runtime, not the operating system.
- 3
Explain that goroutines are more efficient than threads in terms of memory and performance.
- 4
State that goroutines use channels for communication, simplifying concurrency.
- 5
Contrast them with threads by discussing their creation and management overhead.
Example Answers
Goroutines are lightweight functions that run concurrently in Go. They are managed by the Go runtime, making them more efficient than OS threads. While threads consume more resources and have more overhead, goroutines can easily scale with many concurrent tasks. Communication between them is typically done using channels.
How does Go manage memory, and what tools can you use to monitor memory usage?
How to Answer
- 1
Explain Go's garbage collection mechanism briefly
- 2
Highlight the role of the stack and heap in memory management
- 3
Mention tools like pprof, trace, and runtime metrics
- 4
Discuss the importance of profiling and analyzing memory usage
- 5
Suggest practices for optimizing memory in Go applications
Example Answers
Go manages memory through automatic garbage collection, which frees up unused memory. It uses a combination of stack and heap allocation for managing memory. Tools like pprof can help profile memory usage and identify bottlenecks.
Describe the Go build process and how you would set up continuous integration for a Go project.
How to Answer
- 1
Explain the Go build process including 'go build', 'go test', and dependency management.
- 2
Mention how to organize Go code in a project structure.
- 3
Discuss using CI tools like GitHub Actions, Travis CI, or CircleCI.
- 4
Highlight the importance of running tests and static analysis in CI.
- 5
End with how to automate deployment steps.
Example Answers
The Go build process starts with using 'go build' to compile the code. I would organize the code in a standard layout, separating packages. For CI, I would use GitHub Actions to run 'go test' and 'golangci-lint' for code quality on every push. Finally, I would automate deployment once tests pass.
Don't Just Read Gopher Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Gopher interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Behavioral Interview Questions
Can you describe a time when you had to quickly adapt to a new environment or system at work? How did you handle it?
How to Answer
- 1
Identify a specific instance where adaptation was required
- 2
Describe the new environment or system concisely
- 3
Explain your thought process and actions taken
- 4
Highlight the outcome or what you learned from the experience
- 5
Keep it positive, focusing on your flexibility and problem-solving skills
Example Answers
In my last job, our team transitioned to a new project management tool. I took the initiative to explore its features online before the official training. By the time the training happened, I was already familiar with the basics and helped my teammates get started, which improved our team's overall efficiency by 20%.
Tell me about a successful project you worked on as part of a team. What was your role, and what made the team successful?
How to Answer
- 1
Choose a project where you had a significant impact
- 2
Highlight your specific role and contributions clearly
- 3
Explain how teamwork strengthened the project outcome
- 4
Mention key strategies or tools that helped the team succeed
- 5
Reflect on one major lesson learned from the experience
Example Answers
In my last semester project, I worked on developing a mobile app for managing personal finances. As the team lead, I coordinated tasks and ensured everyone was aligned on our goals. Our success came from daily stand-up meetings, which kept us accountable, and we used Trello to track progress. A major lesson learned was the importance of clear communication in preventing misunderstandings.
Don't Just Read Gopher Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Gopher interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Describe a situation where you had to take the lead on a project. How did you manage the responsibilities and team dynamics?
How to Answer
- 1
Choose a specific project you led and explain your role clearly.
- 2
Highlight how you assigned tasks based on team members' strengths.
- 3
Mention any challenges you faced and how you resolved them.
- 4
Discuss your communication strategies to keep everyone aligned.
- 5
Conclude with the positive outcome of the project.
Example Answers
In my last internship, I led a team of four to develop a new app feature. I assigned tasks based on our skills; I handled the design while another teammate focused on coding. We faced a tight deadline and I organized daily check-ins to address issues quickly. This clear communication kept us on track, and we successfully launched the feature on time, receiving positive feedback from users.
Describe a creative solution you developed to solve a complex problem at work.
How to Answer
- 1
Start with a clear description of the problem you faced.
- 2
Explain the constraints and challenges related to the problem.
- 3
Detail the innovative approach you took to resolve it.
- 4
Highlight the outcome of your solution.
- 5
Connect how this experience enhanced your skills relevant to a Gopher position.
Example Answers
In my previous role, we faced a significant delay in project timelines due to inefficient communication between teams. I proposed implementing a shared project management tool that allowed real-time updates and transparency. This streamlined our workflow, reduced delays by 30%, and improved overall team collaboration.
How do you stay updated with the latest trends and technologies in programming, especially Go?
How to Answer
- 1
Follow Go-related blogs and websites for news and articles.
- 2
Join Go communities on platforms like Reddit and Discord.
- 3
Attend meetups and conferences focused on Go programming.
- 4
Contribute to open-source Go projects on GitHub.
- 5
Read Go programming books and documentation regularly.
Example Answers
I follow several Go blogs and websites like Golang News and the Go Blog to keep up with the latest articles. I also participate in Go communities on Reddit and Discord, which helps me stay informed about trends.
Can you give an example of a complex problem you solved? What was your approach and the outcome?
How to Answer
- 1
Choose a specific and relevant complex problem
- 2
Clearly outline your thought process and approach
- 3
Focus on the actions you took to solve the problem
- 4
Highlight the tools or methods used during the solution
- 5
Conclude with the positive outcome and what you learned
Example Answers
In my last project, we faced a data inconsistency issue that affected reporting accuracy. I analyzed the data flow, identified where discrepancies were introduced, and implemented a validation script to catch errors early. As a result, our reporting was 100% accurate, and I learned the importance of proactive data validation.
Tell me about a time when effective communication led to a successful outcome on a project.
How to Answer
- 1
Choose a specific project where communication was key
- 2
Describe the communication methods used (emails, meetings, etc.)
- 3
Mention the stakeholders involved and their roles
- 4
Explain how communication resolved challenges or misunderstandings
- 5
End with the positive outcome that resulted from effective communication
Example Answers
In my last project, I coordinated a team launch for a new software. I organized regular team meetings to ensure everyone was on the same page, clarified tasks, and addressed any questions. This clear communication among team members helped us successfully launch the project ahead of schedule.
Describe an instance where you identified an opportunity for improvement in your work area and took the initiative to implement it.
How to Answer
- 1
Think of a specific situation where your work process could be enhanced.
- 2
Explain the steps you took to identify the problem.
- 3
Describe the initiative you implemented and its impact.
- 4
Use metrics or feedback to show the improvement if possible.
- 5
Keep the focus on your actions and the results.
Example Answers
In my last internship, I noticed that our team spent a lot of time on manual data entry. I researched automation tools and proposed using a spreadsheet script to speed up the process. After getting approval, I set it up, which reduced our data entry time by 50%.
How have you ensured that your work consistently meets the needs and expectations of clients or stakeholders?
How to Answer
- 1
Always clarify client expectations at the start of a project.
- 2
Regularly check in with clients to gather feedback during the process.
- 3
Adapt your work based on feedback to improve outcomes.
- 4
Document changes made in response to stakeholder input.
- 5
Set measurable goals to assess whether client needs are being met.
Example Answers
In my previous role, I ensured client satisfaction by having a kickoff meeting to clarify expectations. During the project, I held bi-weekly check-ins, which allowed me to adjust our approach based on their feedback.
How do you prioritize your tasks when you have multiple deadlines to meet?
How to Answer
- 1
List all tasks and their deadlines
- 2
Identify which tasks are most critical to project goals
- 3
Estimate the time required for each task
- 4
Consider dependencies between tasks
- 5
Communicate with your team if there is a need to adjust priorities
Example Answers
I start by listing all my tasks along with their deadlines. Then, I evaluate which tasks are critical for the project and estimate the time each will take. If some tasks depend on others, I prioritize those first and communicate with my team if needed.
Don't Just Read Gopher Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Gopher interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Situational Interview Questions
You are asked to optimize a slow-running Go application. What steps would you take to identify and fix the performance issues?
How to Answer
- 1
Use the Go profiler to identify bottlenecks in CPU and memory usage.
- 2
Run benchmarks to understand the performance of specific functions.
- 3
Analyze goroutine usage to check for deadlocks or excessive blocking.
- 4
Evaluate inefficient algorithms or data structures in critical paths.
- 5
Consider adding caching mechanisms to reduce redundant computations.
Example Answers
First, I would use the Go profiler to analyze the CPU and memory usage to identify which functions are consuming the most resources. Then, I'd run some benchmarks to test performance changes. Based on the findings, I would refactor inefficient algorithms or leverage caching for repeated calculations.
You disagree with a team member's approach to solving a problem in Go. How would you handle this situation?
How to Answer
- 1
Stay calm and respectful in your response
- 2
Ask clarifying questions to understand their perspective
- 3
Share your concerns with specific examples from the code
- 4
Suggest alternatives and open the floor for discussion
- 5
Aim for a collaborative approach to reach the best solution
Example Answers
I would first ask my team member to explain their approach in detail. I would listen carefully and then share my concerns, highlighting the specific aspects of their solution that might lead to issues. Then, I would propose an alternative method and invite them to collaborate on refining both ideas.
Don't Just Read Gopher Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Gopher interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Your project requires you to use an external API, but it's rate-limited. How would you design your application to handle this constraint?
How to Answer
- 1
Implement exponential backoff strategy for retries
- 2
Use caching to store responses and limit API calls
- 3
Queue requests that exceed the rate limit
- 4
Monitor and log API usage to avoid hitting limits
- 5
Consider using multiple API keys or endpoints if allowed
Example Answers
I would implement an exponential backoff strategy for handling retries when the API response indicates a rate limit has been reached. This helps to avoid overwhelming the API and respects its constraints.
You are close to a project deadline, but a critical bug is discovered. How would you handle the situation?
How to Answer
- 1
Immediately assess the severity and impact of the bug.
- 2
Communicate with the team and stakeholders about the issue.
- 3
Prioritize fixing the bug based on the deadline and project criticality.
- 4
If possible, implement a temporary workaround.
- 5
Stay calm and focused, and ensure clear documentation of the issue.
Example Answers
I would first assess how critical the bug is and what impact it has on the deadline. Then, I'd inform my team and stakeholders immediately and discuss if we can prioritize fixing it or if we need a temporary solution. I would ensure everything is documented clearly.
A client requests a feature that you believe is not feasible within the current project constraints. How would you address this?
How to Answer
- 1
Acknowledge the client's request and its importance.
- 2
Explain the current project constraints clearly.
- 3
Propose alternative solutions that fulfill their needs.
- 4
Invite further discussion to explore options together.
- 5
Document the conversation for future reference.
Example Answers
I appreciate the client's request for this feature and understand its importance. However, given our current project constraints regarding time and resources, I believe implementing this feature may not be feasible. I can suggest some alternative options that might achieve similar results. Would you like to discuss those?
You've been tasked with reviewing a colleague's Go code and notice several inefficiencies. How would you provide feedback?
How to Answer
- 1
Start by praising the overall structure of the code to establish a positive tone.
- 2
Focus on specific inefficiencies you found, such as long-running loops or excessive memory usage.
- 3
Suggest concrete improvements, like using Go's concurrency features or optimizing algorithms.
- 4
Encourage open discussion by asking how they approached certain decisions.
- 5
Offer to help implement the changes or review any revisions they make.
Example Answers
I really appreciate the structure of your code. I noticed that you're using a nested loop which could be optimized using a map to reduce time complexity. Would you be open to discussing this improvement?
You notice that the scope of your project is expanding beyond the initial requirements. How would you manage this?
How to Answer
- 1
Assess the reasons for scope expansion and identify key changes.
- 2
Communicate with stakeholders to understand their needs and expectations.
- 3
Evaluate the impact of scope changes on the project timeline and resources.
- 4
Document all changes and make necessary adjustments to project plans.
- 5
Consider implementing a change control process to manage future scope adjustments.
Example Answers
I would first analyze why the scope is expanding. Then, I would discuss the changes with stakeholders to ensure their needs are met. Next, I would assess the impact on the timeline and resources, document everything, and adjust the project plan accordingly.
You receive conflicting priorities from two managers. How would you handle this?
How to Answer
- 1
Clarify the priorities with both managers to understand their urgency.
- 2
Assess the impact of each task and communicate any potential conflicts.
- 3
Propose a plan to balance the tasks based on the urgency and importance.
- 4
Keep both managers updated on progress to maintain transparency.
- 5
Seek feedback from both managers to adjust priorities if necessary.
Example Answers
I would first clarify with each manager to understand the reasons behind their priorities. After assessing which task has the greatest impact, I would propose a plan that incorporates both tasks, keeping one manager informed of my progress with the other.
Your team discovered a security vulnerability in a Go application. What actions would you take?
How to Answer
- 1
Identify and assess the impact of the vulnerability immediately
- 2
Notify your team and relevant stakeholders about the issue
- 3
Develop a patch or workaround to mitigate the vulnerability
- 4
Test the fix thoroughly to ensure it resolves the issue without introducing new bugs
- 5
Communicate the fix to users and document the vulnerability and resolution
Example Answers
First, I would assess the severity and impact of the vulnerability to understand the risks. Then, I'd alert my team and stakeholders. Next, I would work on developing a patch while ensuring it's thoroughly tested. Finally, I'd communicate the resolution to our users and document the process for future reference.
You encounter an unexpected obstacle in your current project. How do you proceed?
How to Answer
- 1
Stay calm and assess the situation without panic
- 2
Identify the specific obstacle and gather relevant information
- 3
Consider potential solutions and evaluate their feasibility
- 4
Communicate with your team to gather input and support
- 5
Develop a clear plan of action to address the obstacle
Example Answers
When faced with an unexpected obstacle, I first take a moment to remain calm. I then analyze the issue to understand its impact on the project. Next, I brainstorm possible solutions and discuss them with my team to ensure we're on the same page. Finally, I implement the chosen solution with a focus on moving forward efficiently.
Don't Just Read Gopher Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Gopher interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates