Top 29 Java Programmer Interview Questions and Answers [Updated 2025]
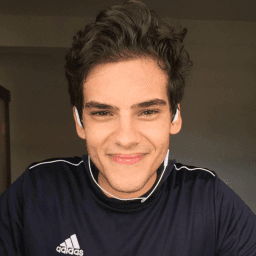
Andre Mendes
•
March 30, 2025
Preparing for a Java Programmer interview can be daunting, but don't worry—this blog post has you covered with the most common interview questions you'll likely encounter. We've compiled not just the questions but also example answers and effective tips to help you stand out. Dive in to boost your confidence and arm yourself with the knowledge to impress your future employer.
Download Java Programmer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Java Programmerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Java Programmer Interview Questions
Behavioral Interview Questions
Can you describe a time when you collaborated on a Java project with your team? What role did you play?
How to Answer
- 1
Choose a specific project and clarify your role clearly
- 2
Highlight your contributions, such as coding, testing, or designing
- 3
Mention how you communicated with team members and resolved conflicts
- 4
Include outcomes or successes of the project to show impact
- 5
Keep it concise and focused on your experience
Example Answers
In a recent project, I worked as a backend developer in a team of five. My main role was to implement RESTful APIs in Java using Spring Boot. I coordinated with the front-end team to ensure seamless data flow and utilized Git for version control, which helped us stay organized. Our project launched on time and improved client data handling by 30%.
Tell me about a challenging bug you encountered in your Java code. How did you approach fixing it?
How to Answer
- 1
Describe the bug clearly and concisely.
- 2
Explain the investigation process you used to identify the root cause.
- 3
Discuss the steps you took to fix the bug.
- 4
Mention any tools or methods that were particularly helpful.
- 5
Reflect on what you learned from the experience.
Example Answers
I encountered a NullPointerException when accessing an object in my Java application. I used debugging tools to trace the code execution. I found that the object wasn't initialized properly due to a race condition. I implemented synchronization to ensure safe access, and the bug was resolved. This taught me the importance of thread safety in Java.
Don't Just Read Java Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Have you ever led a project or a team of developers? What was your approach to managing the team?
How to Answer
- 1
Highlight a specific project you led
- 2
Explain your leadership style and how it helped the team
- 3
Mention tools or methodologies you used to manage tasks
- 4
Discuss how you handled challenges or conflicts within the team
- 5
Talk about the results or successes achieved through your leadership
Example Answers
In my last project, I led a team of 5 developers. I adopted an agile approach, holding daily stand-ups to track progress and address issues quickly. This improved our workflow and reduced bottlenecks, leading to a successful launch on time.
Describe a situation where you had to learn a new technology or framework quickly. How did you manage that?
How to Answer
- 1
Choose a specific example that illustrates your adaptability.
- 2
Outline the steps you took to learn the technology, including resources used.
- 3
Discuss any challenges faced and how you overcame them.
- 4
Highlight the outcomes or results of your learning experience.
- 5
Keep it brief and focused on your actions and results.
Example Answers
In my previous job, I had to learn Spring Boot for a new project. I dedicated a weekend to reading the official documentation and followed a tutorial to build a small application. The challenge was understanding dependency injection, but I practiced by experimenting with different configurations. As a result, I successfully integrated Spring Boot in our project, leading to a faster development cycle.
Can you give me an example of how you explained a complex Java concept to a non-technical stakeholder?
How to Answer
- 1
Identify the complex concept clearly
- 2
Use simple language without jargon
- 3
Relate the concept to everyday experiences
- 4
Encourage questions to ensure understanding
- 5
Summarize key points at the end
Example Answers
I explained polymorphism in Java by comparing it to how a single remote control can operate different devices. I used everyday examples to clarify how one interface can be used to interact with various classes.
Describe a time you received constructive criticism on your code. How did you handle it?
How to Answer
- 1
Be specific about the feedback you received.
- 2
Explain your initial reaction to the criticism.
- 3
Describe the steps you took to improve your code.
- 4
Share the outcome of implementing the feedback.
- 5
Reflect on what you learned from the experience.
Example Answers
During a code review, my colleague pointed out that my method was too complex and suggested breaking it down into smaller functions. Initially, I felt defensive but realized the value in that suggestion. I refactored the code, which made it cleaner and easier to maintain. The project improved in readability, and I learned the importance of simplicity in coding.
Discuss a long-term goal you set for your career as a Java programmer. How did you work towards achieving it?
How to Answer
- 1
Identify a specific long-term goal related to Java programming.
- 2
Explain the steps you took to reach that goal.
- 3
Mention any skills you developed or certifications you pursued.
- 4
Describe any projects or experiences that contributed to your growth.
- 5
Highlight how this goal aligns with your overall career aspirations.
Example Answers
My long-term goal is to become a lead Java developer. To achieve this, I enrolled in advanced Java courses, actively contributed to open-source projects, and sought mentorship from senior developers. These steps helped me enhance my coding skills and take on leadership roles in team projects.
Technical Interview Questions
What are the main differences between Java 8 and previous versions? Can you explain some key features introduced in Java 8?
How to Answer
- 1
Start by mentioning the overall shift towards functional programming.
- 2
Highlight key features like Lambda expressions and Streams API.
- 3
Discuss the introduction of the Optional class to handle nulls.
- 4
Mention enhancements to the Date and Time API.
- 5
Conclude with the impact these features have on code readability and efficiency.
Example Answers
Java 8 marked a significant shift towards functional programming with features like Lambda expressions, which allow for more concise code. The Streams API introduced a new way to process collections, making data manipulation easier and more intuitive. Additionally, the Optional class helps prevent null pointer exceptions, enhancing code safety.
How do you implement polymorphism in Java? Can you provide a code example?
How to Answer
- 1
Define polymorphism as the ability of an object to take many forms.
- 2
Mention the two types: compile-time (method overloading) and runtime (method overriding).
- 3
Use a clear code example that illustrates runtime polymorphism with a superclass and subclass.
- 4
Explain how the method in the subclass overrides the one in the superclass.
- 5
Keep the example simple to demonstrate understanding without unnecessary complexity.
Example Answers
Polymorphism in Java allows methods to do different things based on the object that is calling them. For example, using method overriding, a superclass 'Animal' can have a method 'sound'. A subclass 'Dog' can override this method to provide a specific implementation. Here’s a brief code snippet: class Animal { void sound() { System.out.println('Animal makes a sound'); } } class Dog extends Animal { void sound() { System.out.println('Bark'); } } Animal myDog = new Dog(); myDog.sound(); // This will print 'Bark'.
Don't Just Read Java Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What techniques do you use to optimize the performance of a Java application?
How to Answer
- 1
Identify and eliminate memory leaks by analyzing the heap and using profiling tools
- 2
Optimize algorithms and data structures for efficiency based on use cases
- 3
Minimize synchronization in multi-threaded applications to reduce contention
- 4
Use caching mechanisms like Ehcache or Redis to store frequently accessed data
- 5
Monitor and fine-tune garbage collection settings to reduce pause times
Example Answers
I often start by profiling the application to find memory leaks, which I address using appropriate tools. Then, I optimize algorithms to ensure they are the most efficient for the data at hand.
Explain the difference between checked and unchecked exceptions in Java. When should each be used?
How to Answer
- 1
Define checked exceptions as those that must be declared or handled.
- 2
Define unchecked exceptions as those that do not require explicit handling.
- 3
Provide examples of each type, like IOException for checked and NullPointerException for unchecked.
- 4
Explain use cases where checked exceptions represent recoverable conditions.
- 5
Discuss how unchecked exceptions often indicate programming errors.
Example Answers
Checked exceptions require handling by the programmer, like IOException, which indicates a recoverable error. Unchecked exceptions, like NullPointerException, usually suggest bugs in code. Use checked exceptions for issues that can be anticipated and managed.
Which Java collections do you prefer to use for specific scenarios and why?
How to Answer
- 1
Identify the specific scenario you are discussing.
- 2
Choose a collection type that best fits the scenario's requirements.
- 3
Explain your choice with characteristics of the collection.
- 4
Mention performance considerations where applicable.
- 5
Provide a brief example of usage for clarity.
Example Answers
For a scenario where I need to maintain unique elements, I prefer using HashSet because it offers O(1) time complexity for add and contains operations. For example, I use HashSet to store user IDs to prevent duplicates in my application.
What is your approach to writing unit tests for Java applications? Do you use any specific frameworks?
How to Answer
- 1
Focus on the importance of unit tests in maintaining code quality.
- 2
Mention specific testing frameworks you are familiar with, like JUnit or Mockito.
- 3
Explain your process for identifying what to test, such as edge cases and business logic.
- 4
Discuss how you organize tests and keep them maintainable.
- 5
Emphasize running tests frequently, especially during development and before releases.
Example Answers
I believe unit tests are crucial for ensuring code quality and catching issues early. I primarily use JUnit for writing my tests, focusing on key areas like edge cases and business logic. I keep my tests organized in packages reflecting the main code structure, and I run them regularly during development to ensure everything works correctly.
Can you explain how threading works in Java? Include the differences between processes and threads.
How to Answer
- 1
Start by defining what a thread is and how it's a lightweight process.
- 2
Explain Java's threading model using the Thread class and Runnable interface.
- 3
Mention common threading concepts like synchronization and blocking.
- 4
Clarify the difference between processes and threads, especially in terms of resource usage.
- 5
Conclude with practical use cases for threads in Java applications.
Example Answers
In Java, a thread is a lightweight unit of execution within a process, allowing for concurrent operations. We use the Thread class or implement the Runnable interface to create threads. Unlike processes, threads share the same memory space which leads to less resource consumption. However, this requires careful management to avoid issues like race conditions.
What security practices do you implement when writing Java applications, especially for web-based applications?
How to Answer
- 1
Use prepared statements to prevent SQL injection.
- 2
Implement input validation and sanitization to protect against XSS.
- 3
Utilize HTTPS to secure data in transit.
- 4
Apply proper authentication and authorization checks.
- 5
Regularly update libraries and frameworks to patch vulnerabilities.
Example Answers
I ensure to use prepared statements to avoid SQL injection and always validate user inputs to protect against XSS attacks.
Which Java frameworks have you worked with? Can you compare Spring vs. Hibernate in terms of functionality?
How to Answer
- 1
List specific frameworks you have experience with, focusing on Java-related ones.
- 2
Focus on the primary functions of Spring and Hibernate during comparison.
- 3
Mention use cases or scenarios where each framework excels.
- 4
Emphasize how they complement each other in a typical application.
- 5
Be prepared to discuss personal projects or roles involving these frameworks.
Example Answers
I have worked extensively with Spring and Hibernate. Spring is primarily a framework for building enterprise applications, providing features like dependency injection and aspect-oriented programming. Hibernate, on the other hand, is a powerful ORM tool for managing database operations. In projects, I've used Spring for the overall application structure and Hibernate for data persistence, ensuring efficient and robust database interactions.
How do you build RESTful APIs in Java? What tools do you use for this?
How to Answer
- 1
Define key principles of RESTful APIs, such as statelessness and resource identification.
- 2
Mention the use of frameworks like Spring Boot for rapid development.
- 3
Highlight the importance of using annotations for mapping requests to Java methods.
- 4
Discuss tools for testing APIs, like Postman or JUnit.
- 5
Refer to documentation for API development and best practices.
Example Answers
To build RESTful APIs in Java, I typically use Spring Boot for its convenience. I define resources using annotations like @RestController and @RequestMapping. For testing, I rely on Postman to verify endpoints and JUnit for automated testing.
Don't Just Read Java Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
How do you handle dependency management in Java projects? What tools do you prefer to use?
How to Answer
- 1
Discuss the importance of dependency management for project stability.
- 2
Mention specific tools like Maven or Gradle and their benefits.
- 3
Explain how to handle versioning and transitive dependencies.
- 4
Share a personal experience of resolving a dependency issue.
- 5
Highlight the role of dependency management in team collaboration.
Example Answers
In my Java projects, I use Maven for dependency management because it simplifies handling library versions and transitive dependencies. For example, I recently resolved a conflict by updating the version in the POM file, allowing the entire team to build without issues.
Situational Interview Questions
If you were given a legacy Java application that frequently crashes, what steps would you take to diagnose and fix the issues?
How to Answer
- 1
Check application logs for error messages or stack traces
- 2
Identify specific scenarios that trigger the crashes
- 3
Use a debugger to step through the code during execution
- 4
Analyze resource usage like memory and CPU while the app runs
- 5
Review recent code changes or dependencies that may have introduced issues
Example Answers
First, I would analyze the application logs to find relevant error messages that indicate what is causing the crashes. Then, I would replicate the scenarios that lead to the crashes to pinpoint issues. Afterward, I might use a debugger to trace through the code and check for any logical errors.
Imagine you are asked to design a new feature for an application using a specific design pattern. Which pattern would you choose and why?
How to Answer
- 1
Identify the feature requirements clearly
- 2
Choose a design pattern that solves specific problems
- 3
Explain your choice with real-world examples
- 4
Discuss the advantages of the selected pattern
- 5
Be ready to mention potential drawbacks or alternatives
Example Answers
I would use the Observer pattern to implement a notification system for users. It allows multiple components to receive updates when changes occur, which is essential for real-time communication.
Don't Just Read Java Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
How would you handle a situation where you found critical issues in a teammate's Java code during a code review?
How to Answer
- 1
Start with a positive tone, acknowledging the teammate's effort.
- 2
Be specific about the issues you've found, providing examples.
- 3
Suggest potential solutions or improvements rather than just pointing out problems.
- 4
Facilitate a collaborative discussion to address the issues together.
- 5
Focus on learning and growth rather than blame.
Example Answers
I would begin by appreciating the team's hard work and then point out the critical issues with specific examples. For instance, I would say, 'I noticed some null pointer exceptions in this method; we can handle those by adding proper checks. What do you think?' This way, we can work together to improve the code.
If you're approaching a deadline for a Java project, but realize that you won't meet it with your current progress, what would you do?
How to Answer
- 1
Assess the remaining tasks and prioritize them
- 2
Communicate with your team or manager about the situation
- 3
Explore options for reducing scope or features
- 4
Consider reallocating resources or getting help
- 5
Set a new plan with deadlines for the prioritized tasks
Example Answers
First, I would assess my current tasks and prioritize the critical features that need to be completed. Next, I would communicate with my team to discuss the situation and see if we can adjust the project scope. If necessary, I would consider asking for assistance from colleagues.
How would you approach integrating a third-party Java library into an existing application?
How to Answer
- 1
Identify the specific functionality needed from the library
- 2
Check for compatibility with the existing application version
- 3
Read the library documentation for integration steps
- 4
Add the library to the project's build system (e.g., Maven, Gradle)
- 5
Test the integration thoroughly to ensure it works as expected
Example Answers
First, I would determine the specific functionality I need from the library. Next, I'd verify that the library is compatible with my application's Java version. Then, I would consult the library documentation for integration guidelines. After that, I'd add the library to my build system, such as using Maven or Gradle, and finally, I would run tests to ensure everything integrates smoothly.
Imagine you've been asked to scale an existing Java application to handle increased traffic. What would be your first steps?
How to Answer
- 1
Analyze current performance metrics to identify bottlenecks
- 2
Optimize the existing codebase for efficiency and speed
- 3
Implement caching strategies to reduce load on server resources
- 4
Consider adding load balancers to distribute traffic effectively
- 5
Scale horizontally by adding more instances or services
Example Answers
First, I would analyze performance metrics to pinpoint bottlenecks. Then, I'd optimize the code to improve response times. Implementing caching strategies would be next to reduce server load. After that, I would explore using load balancers to distribute incoming traffic evenly. Finally, scaling horizontally by adding more instances would help accommodate increased traffic.
If you receive unclear requirements for a new Java feature, how would you ensure you gather the correct information?
How to Answer
- 1
Ask clarifying questions to understand the main goals.
- 2
Request examples or use cases to provide context.
- 3
Engage stakeholders to verify assumptions and needs.
- 4
Document the requirements discussed for accuracy.
- 5
Follow up with a summary email to confirm understanding.
Example Answers
I would start by asking specific questions to clarify the main objectives and desired outcomes. Then, I would request examples to visualize the functionality, ensuring I capture all necessary details.
How would you approach modifying a legacy Java application that lacks documentation? What steps would you take?
How to Answer
- 1
Start by reviewing the existing code to understand its structure and functionality.
- 2
Run existing tests or create new ones to ensure you understand current behavior.
- 3
Identify key components and their dependencies through code analysis tools.
- 4
Use version control to document changes and keep track of modifications.
- 5
Engage with any stakeholders or users to gather insights about needed features.
Example Answers
I would begin by exploring the codebase thoroughly, looking for key functionalities and structures. Running any existing tests would help clarify how the application behaves. I'd also identify dependencies using tools like JDepend, and document my modifications through commits in version control. Finally, I'd consult with users to gather their input on necessary changes.
If a client wants a Java application to have functionality that you believe is unnecessary, how would you handle that discussion?
How to Answer
- 1
Listen actively to understand the client's reasoning and needs.
- 2
Acknowledge the client's perspective before sharing your concerns.
- 3
Present data or examples to support your viewpoint on the functionality.
- 4
Suggest alternative solutions that align with the client's goals.
- 5
Maintain professionalism and be open to collaboration.
Example Answers
I would first listen carefully to the client's reasons for wanting the functionality. Then, I would share my concerns and the potential drawbacks, backed by data or examples. I would also propose an alternative approach that could achieve their goals more efficiently.
What would you do if you realized that a project is falling behind schedule because of a lack of collaboration among team members?
How to Answer
- 1
Identify the specific collaboration issues causing delays
- 2
Schedule a team meeting to discuss challenges openly
- 3
Encourage team members to share their progress and roadblocks
- 4
Facilitate a brainstorming session to find solutions together
- 5
Set clear roles and responsibilities to enhance accountability
Example Answers
I would first identify the areas where collaboration is lacking and then schedule a meeting to address these issues. During the meeting, I would encourage each team member to share their progress and any roadblocks they face, fostering open communication.
Don't Just Read Java Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Java Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Java Programmer Position Details
Recommended Job Boards
These job boards are ranked by relevance for this position.
Related Positions
- Java Developer
- Software Programmer
- .NET Programmer
- Web Programmer
- Java Architect
- Computer Programmer
- Game Programmer
- Database Programmer
- Application Programmer
- Programmer
Similar positions you might be interested in.
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates