Top 30 Application Programmer Interview Questions and Answers [Updated 2025]
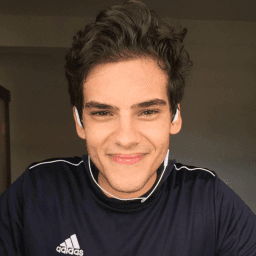
Andre Mendes
•
March 30, 2025
Preparing for an application programmer interview can be daunting, but we're here to help streamline your preparation process. In this post, we've compiled the most common interview questions you might encounter for this role. With example answers and practical tips on how to respond effectively, you'll gain the confidence and insights needed to impress potential employers and ace your interview. Dive in and get ready to succeed!
Download Application Programmer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Application Programmerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Application Programmer Interview Questions
Technical Interview Questions
How do you decide which programming framework or library to use for a project?
How to Answer
- 1
Evaluate the project requirements and specifications first
- 2
Consider the team's familiarity and expertise with different frameworks
- 3
Research community support and documentation for the frameworks
- 4
Assess the performance and scalability needs of the project
- 5
Think about the maintenance and long-term viability of the framework
Example Answers
I start by analyzing the project requirements, then I look at frameworks that meet those needs while considering my team's familiarity with them. For instance, if we're building a web app, I'd consider React or Angular based on our past experience.
What is the difference between a stack and a queue, and can you provide a practical use case for each?
How to Answer
- 1
Define a stack and a queue clearly
- 2
Explain the main operational difference: LIFO for stack and FIFO for queue
- 3
Provide a real-world example for each data structure
- 4
Keep your explanations concise and focused
- 5
Be prepared to explain why you would choose one structure over the other in a scenario
Example Answers
A stack is a data structure that follows Last In First Out (LIFO) while a queue follows First In First Out (FIFO). For example, a practical use case for a stack is in function call management in programming languages, where the last function called is the first one to return. A queue's practical use case is in processing tasks in order, like customer service lines, where the first customer to arrive is the first to be served.
Don't Just Read Application Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Application Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What are design patterns, and can you give an example of when you have used one in your application development?
How to Answer
- 1
Define design patterns briefly and mention their purpose in software design.
- 2
Select a specific design pattern you are familiar with, like Singleton or Factory.
- 3
Describe a project where you implemented that pattern and the problem it solved.
- 4
Relate the benefits of using the design pattern in your project.
- 5
Keep your answer concise and focused on your personal experience.
Example Answers
Design patterns are best practices that help solve common design problems. I used the Singleton pattern in a project where I needed to ensure only one instance of a database connection was created. This helped manage resources efficiently and improved performance.
Explain the process of normalizing a database and why it is important.
How to Answer
- 1
Define normalization and its purpose in organizing data.
- 2
Describe the different normal forms, emphasizing the first three (1NF, 2NF, 3NF).
- 3
Explain how normalization reduces redundancy and improves data integrity.
- 4
Discuss the implications of normalization on database efficiency and performance.
- 5
Mention scenarios where denormalization might be considered despite its downsides.
Example Answers
Normalization is a process in database design to reduce redundancy and improve data integrity. It involves organizing data into tables and ensuring that each table follows the rules of normal forms. For example, the first normal form requires that all entries in a column be atomic and unique. This helps streamline updates and maintain consistent data.
What are the four main concepts in object-oriented programming, and how do they benefit software development?
How to Answer
- 1
Define the four main concepts: encapsulation, inheritance, polymorphism, and abstraction.
- 2
Explain each concept briefly and its role in OOP.
- 3
Highlight how these concepts improve code maintainability and reuse.
- 4
Mention real-world examples or scenarios where OOP benefits are evident.
- 5
Keep your answer focused on their practical advantages in software development.
Example Answers
The four main concepts of OOP are encapsulation, inheritance, polymorphism, and abstraction. Encapsulation allows us to bundle data and methods together, improving code organization and security. Inheritance enables code reuse and hierarchical classification. Polymorphism offers flexibility, allowing methods to do different things based on the object they are invoked on. Lastly, abstraction helps manage complexity by hiding unnecessary details. Together, they enhance code maintainability and scalability.
What is RESTful API design? How do you ensure that an API is scalable and maintainable?
How to Answer
- 1
Define RESTful API design principles clearly
- 2
Mention key concepts like statelessness, resource-based, and use of HTTP methods
- 3
Discuss versioning and documentation for maintainability
- 4
Explain how to use caching and load balancing for scalability
- 5
Highlight the importance of clean versioning and consistent endpoint management
Example Answers
RESTful API design focuses on using standard HTTP methods like GET, POST, PUT, and DELETE for different operations on resources. To ensure scalability, I implement caching strategies and load balancing. For maintainability, I keep thorough documentation and version the API intelligently so that changes can be made without breaking existing users.
How do you approach writing unit tests, and why are they important?
How to Answer
- 1
Start by understanding the functionality you want to test
- 2
Write tests for individual functions or methods focusing on specific outcomes
- 3
Use a testing framework suitable for your programming language
- 4
Keep tests independent and avoid dependencies on external systems
- 5
Emphasize the value of tests in maintaining code quality and catching bugs early
Example Answers
I approach writing unit tests by first identifying the critical functions in my code that require testing. I use a framework like JUnit for Java, and I write tests that assert expected outcomes based on various inputs. Unit tests are essential because they help me catch errors early and ensure that changes or new features don’t break existing functionality.
What techniques do you use to optimize the performance of an application?
How to Answer
- 1
Identify bottlenecks using profiling tools
- 2
Optimize algorithms and data structures
- 3
Reduce memory usage and manage resources efficiently
- 4
Implement caching strategies for repetitive tasks
- 5
Utilize asynchronous processing where applicable
Example Answers
I use profiling tools like VisualVM to identify bottlenecks, and then I optimize algorithms to reduce time complexity.
What are the advantages of using version control systems like Git, and how do you handle branching and merging in your projects?
How to Answer
- 1
Start by mentioning the fundamental advantages of version control like collaboration, backup, and history tracking.
- 2
Explain specific features of Git that enhance development, such as branching, merging, and version tracking.
- 3
Discuss your personal workflow with branching and merging to provide a practical example.
- 4
Include how you resolve conflicts during merging and keep the codebase stable.
- 5
Mention the benefits of using pull requests and code reviews in your process.
Example Answers
Using version control systems like Git allows teams to collaborate efficiently. It provides a complete history of changes, enabling easy rollback if issues arise. In my projects, I utilize branching to work on features independently. When merging, I ensure to resolve conflicts using Git's tools to maintain a clean history.
Can you explain the benefits of using a hash table and give an example of its use in application development?
How to Answer
- 1
Highlight the speed of access, typically O(1) for lookups.
- 2
Mention how hash tables efficiently handle large datasets.
- 3
Explain collision resolution methods briefly, like chaining or open addressing.
- 4
Provide a concrete example from application development, such as caching or user session management.
- 5
Keep your explanation clear and relate the benefits to real-world scenarios.
Example Answers
Hash tables provide O(1) access time for lookups, which is efficient for large datasets. For example, in a web application, we could use a hash table to cache user sessions, allowing quick retrieval of user data without re-fetching from the database.
Don't Just Read Application Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Application Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Behavioral Interview Questions
Can you describe a time when you successfully collaborated with a team to complete a programming project?
How to Answer
- 1
Choose a specific project and explain its goal
- 2
Describe your role and contributions clearly
- 3
Mention tools or technologies used for collaboration
- 4
Highlight how you resolved challenges as a team
- 5
Conclude with the outcome or success of the project
Example Answers
In my last position, we developed a web application for tracking inventory. I was responsible for the backend logic using Node.js. We used Git for version control and held daily standups to discuss progress. A challenge was integrating with a third-party API, but we brainstormed solutions as a team and implemented a successful workaround. We completed the project on time and improved our client's inventory management by 40%.
Tell me about a time you had a disagreement with a colleague over a technical approach. How did you resolve it?
How to Answer
- 1
Choose a specific example clearly illustrating the disagreement.
- 2
Describe your colleague's viewpoint and your viewpoint without blame.
- 3
Explain how you used data or research to back your approach.
- 4
Talk about the process of discussion and compromise you went through.
- 5
Highlight the positive outcome and what you learned.
Example Answers
I once disagreed with a colleague on using a relational database for a new feature. I believed a NoSQL approach was better due to the expected data volume. We both presented our cases using project data, and I suggested a prototype to test both methods. After analyzing the results together, we found that my approach was indeed more efficient for our needs, and we implemented it successfully.
Don't Just Read Application Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Application Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Describe a situation where you had to quickly learn a new technology or programming language to complete a project. How did you go about it?
How to Answer
- 1
Identify a specific project where quick learning was essential.
- 2
Explain the technology or language needed and why it was important.
- 3
Describe the steps you took to learn it quickly, such as online courses or documentation.
- 4
Share any resources you found particularly helpful.
- 5
Conclude with the outcome of the project and how your learning benefited the team.
Example Answers
In my last job, I had to learn Python on short notice for a data analysis project. I enrolled in an intensive online course and focused on the basics through hands-on practice. I used documentation and built small scripts to test my knowledge. As a result, I completed the project successfully, and our team was able to deliver insights that improved decision-making.
Can you give an example of a time when you introduced a new technology or tool to your team? What was the outcome?
How to Answer
- 1
Choose a specific tool or technology you introduced to the team
- 2
Explain the problem or need that prompted the introduction
- 3
Describe how you implemented the new technology within the team
- 4
Discuss the impact it had on the team's performance or workflow
- 5
End with a takeaway or lesson learned from the experience
Example Answers
I introduced Git as our version control system to the team because we were struggling with code collaboration. I organized a workshop to train everyone, and within weeks, our development process became much smoother, reducing merge conflicts by 60%. The team appreciated the increased transparency and control over code versions.
Tell me about a time when you had limited resources to complete a project. How did you manage to succeed?
How to Answer
- 1
Identify the constraints clearly
- 2
Highlight your problem-solving skills
- 3
Discuss how you prioritized tasks
- 4
Mention any creative solutions or workarounds
- 5
Reflect on the outcome and what you learned
Example Answers
In my previous role, I was assigned a project with a tight deadline and only two team members. I prioritized the key features and divided tasks based on our strengths. I also utilized open-source libraries to speed up development. As a result, we completed the project on time and received positive feedback from our client.
Describe an experience where you helped mentor another team member or peer in programming. What was the impact?
How to Answer
- 1
Choose a specific mentoring experience to discuss
- 2
Focus on the techniques you used to help your peer
- 3
Mention the skills or knowledge they gained
- 4
Explain the positive outcome for both them and the team
- 5
Keep it concise and highlight your role in the process
Example Answers
I mentored a junior developer who was struggling with understanding object-oriented programming. I organized weekly pair programming sessions and shared resources. As a result, they successfully completed their project and improved their coding skills significantly, which also helped the team meet its deadlines.
Give an example of a significant change in a project's direction and how you adapted to it.
How to Answer
- 1
Choose a specific project where you faced a change.
- 2
Explain the nature of the change clearly.
- 3
Detail how you adapted to the new direction.
- 4
Highlight any skills or strategies you used during the adaptation.
- 5
Summarize the positive outcome of your adaptation.
Example Answers
In a project for developing an e-commerce site, the client shifted focus from B2C to B2B functionalities. I quickly gathered requirements from stakeholders, redesigned the data model, and implemented new features like bulk ordering. This adaptability helped us deliver the project on time and meet the client's new goals.
Describe a difficult programming problem you faced and how you solved it.
How to Answer
- 1
Choose a specific problem you've encountered on a project.
- 2
Explain the context and the challenges involved.
- 3
Describe the steps you took to analyze and resolve the issue.
- 4
Highlight any tools or techniques you used.
- 5
Conclude with the outcome and what you learned from the experience.
Example Answers
In my previous job, I encountered a memory leak in a Java application. After isolating the issue using a profiler, I realized that an object was being held in a static collection. I refactored the code to remove the object when it was no longer needed. This reduced the memory usage significantly and improved application performance.
Can you provide an example of how you managed your time effectively during a complex programming project?
How to Answer
- 1
Break down the project into smaller tasks
- 2
Set specific deadlines for each task
- 3
Use project management tools to track progress
- 4
Prioritize tasks based on their impact
- 5
Regularly review and adjust your plan as needed
Example Answers
In my last project, I divided the application into smaller modules and set a deadline for each. I used Trello to track progress and ensured I focused on high-impact features first.
Describe a time when you proactively identified a problem in a project and took the initiative to resolve it.
How to Answer
- 1
Use the STAR method: Situation, Task, Action, Result.
- 2
Choose a specific instance from your past experience.
- 3
Explain how you identified the problem with clear reasoning.
- 4
Detail the steps you took to address the issue.
- 5
Highlight the positive outcome of your actions.
Example Answers
In a recent project, I noticed that our application's performance was degrading as we added features. I conducted a performance audit (Situation), realized that unoptimized queries were the issue (Task). I refactored these queries and implemented caching strategies (Action). As a result, our app's performance improved by 40% and user feedback was overwhelmingly positive (Result).
Don't Just Read Application Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Application Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Situational Interview Questions
Imagine you are assigned a bug to fix in a legacy application with minimal documentation. How would you approach the problem?
How to Answer
- 1
Understand the bug by reproducing it in the application
- 2
Review the existing code and identify where the bug might originate
- 3
Use debugging tools to step through the code execution
- 4
Consult colleagues for insights on the legacy system
- 5
Document your findings and the steps you took to fix the bug
Example Answers
First, I would try to reproduce the bug to see its behavior and understand the context. Then, I would analyze the relevant sections of the codebase, checking for potential issues. Using debugging tools, I would step through the code to find where it deviates from expected behavior. If needed, I would also ask team members for their experiences with this part of the application. Lastly, I would document both the bug and my fix for future reference.
You have a tight deadline for deploying a feature, but you discover a critical bug close to the deadline. What steps would you take to handle the situation?
How to Answer
- 1
Assess the severity of the bug and its impact on the feature.
- 2
Communicate the discovery of the bug to the relevant stakeholders immediately.
- 3
Prioritize fixing the bug over adding new features.
- 4
Determine if a temporary workaround is possible for the deployment.
- 5
If necessary, consider negotiating the deadline based on the criticality of the issue.
Example Answers
First, I would evaluate how critical the bug is and its potential impact on users. Then, I would inform my team and stakeholders right away. I’d focus on fixing the bug instead of adding new features, and if feasible, I would explore a temporary workaround to ensure we meet the deadline.
Don't Just Read Application Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Application Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
A client has requested a feature that you believe is technically challenging to implement. How would you handle this request?
How to Answer
- 1
Acknowledge the client's request and express appreciation for their input
- 2
Assess the technical challenges and identify specific issues
- 3
Communicate openly with the client about potential difficulties
- 4
Suggest alternative solutions that could meet their needs
- 5
Propose a phased approach for implementation if necessary
Example Answers
I would start by thanking the client for their request and clarify the feature's importance. Then, I would analyze the challenges involved and communicate transparently about any technical hurdles we might encounter. I could propose a simpler alternative that achieves similar results, or suggest breaking the feature into phases for gradual implementation.
You receive feedback that your code lacks clarity and structure. How would you address this feedback and improve your coding practices?
How to Answer
- 1
Reflect on the specific areas where the code was unclear.
- 2
Incorporate meaningful comments that explain complex logic.
- 3
Use consistent naming conventions for variables and functions.
- 4
Break down large functions into smaller, reusable components.
- 5
Review and refactor code regularly to enhance clarity.
Example Answers
I would analyze the feedback to identify unclear sections, then I would add comments explaining the logic and ensure that my variable names are descriptive. Additionally, I would refactor my code into smaller functions to improve readability.
A new feature is requested that requires significant changes to the system architecture. What factors would you consider before implementing it?
How to Answer
- 1
Evaluate the impact on existing system performance and stability
- 2
Consider the time and resources required for implementation
- 3
Assess how the change aligns with the overall product roadmap
- 4
Identify potential risks and how to mitigate them
- 5
Engage stakeholders for feedback and approval
Example Answers
I would first assess how the new feature might affect the system's current performance and stability, ensuring that any implementation won't disrupt existing services. Next, I'd consider the amount of developer resources and time needed for this change, comparing it to our project timelines. Aligning the feature with our overall product roadmap is critical to maintain strategic focus. I would also identify any risks involved with the changes and think of mitigation strategies. Lastly, I would communicate with stakeholders to gather their input and get buy-in before proceeding.
How would you explain a complex technical concept to a non-technical stakeholder?
How to Answer
- 1
Identify the core idea of the concept.
- 2
Use analogies that relate to everyday experiences.
- 3
Avoid technical jargon and use simple language.
- 4
Provide a clear, concise example demonstrating the concept.
- 5
Ask if they have questions or need clarification.
Example Answers
To explain cloud computing, I would say it's like using electricity. Just as you don’t need a generator at home; you can simply plug in and use power when needed, cloud computing lets you use software and storage without having to own servers.
You discover a security vulnerability in your application. What steps would you take to address it?
How to Answer
- 1
Immediately assess the severity of the vulnerability.
- 2
Inform your team and relevant stakeholders about the issue.
- 3
Develop a fix or patch for the vulnerability as quickly as possible.
- 4
Test the fix thoroughly to ensure it resolves the issue without causing new problems.
- 5
Communicate to users if they need to take any action or if their data is at risk.
Example Answers
First, I would assess the severity of the vulnerability to understand the risk it poses. Then, I would inform my team and stakeholders to ensure everyone is aware. Next, I would work on a patch to fix the issue and test it to make sure it works correctly. Finally, I would communicate with our users if any action is required on their part.
You receive negative feedback on a feature from users. How would you handle it, and what steps would you take to improve the feature?
How to Answer
- 1
Acknowledge the feedback and don't take it personally
- 2
Gather specific details from users to understand the issues
- 3
Prioritize changes based on user impact and feasibility
- 4
Implement incremental improvements and test with users iteratively
- 5
Communicate back to users about how their feedback is being used
Example Answers
I would first acknowledge the feedback and reach out to users for specific details on what they found unsatisfactory. Then, I would prioritize the issues based on user impact and start working on the most critical areas. After making changes, I would test the feature with users to ensure improvement and communicate updates to them.
You need to work with a UX designer and a product manager to implement a new feature. How would you ensure effective collaboration among team members?
How to Answer
- 1
Schedule regular check-in meetings to discuss progress and challenges
- 2
Use collaborative tools like Trello or JIRA for task management
- 3
Encourage open communication through shared channels like Slack or Microsoft Teams
- 4
Define clear roles and responsibilities from the start
- 5
Gather and incorporate feedback iteratively throughout the development process
Example Answers
I would set up weekly check-ins to align on our progress and address any roadblocks. Using a tool like JIRA would help us keep track of tasks and responsibilities clearly.
If you are working on multiple projects with competing deadlines, how would you prioritize your work?
How to Answer
- 1
List all projects and their deadlines
- 2
Assess the impact of each project on the business
- 3
Communicate with stakeholders for clarity on priorities
- 4
Break tasks into smaller actionable steps
- 5
Review and adjust priorities as deadlines approach
Example Answers
I would start by creating a list of all my current projects along with their respective deadlines and required deliverables. I would then evaluate which project has the highest business impact and focus on that first, while keeping my team updated on progress and any potential hurdles.
Don't Just Read Application Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Application Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Application Programmer Position Details
Recommended Job Boards
CareerBuilder
www.careerbuilder.com/jobs/application-programmerZipRecruiter
www.ziprecruiter.com/Jobs/Application-ProgrammerThese job boards are ranked by relevance for this position.
Related Positions
- Software Programmer
- Database Programmer
- Game Programmer
- .NET Programmer
- Web Programmer
- Java Programmer
- Systems Programmer
- Programmer
- Computer Programmer
- Website Programmer
Similar positions you might be interested in.
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates