Top 29 Systems Programmer Interview Questions and Answers [Updated 2025]
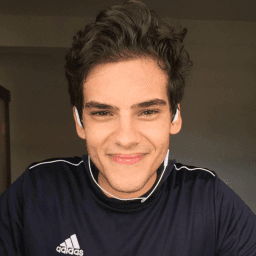
Andre Mendes
•
March 30, 2025
Navigating the competitive landscape of systems programming requires not just technical prowess but also the ability to communicate your skills effectively in an interview. In this blog post, we delve into the most common systems programmer interview questions, providing insightful example answers and practical tips to help you respond with confidence. Prepare to impress potential employers and secure your dream role with our comprehensive guide.
Download Systems Programmer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Systems Programmerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Systems Programmer Interview Questions
Technical Interview Questions
What tools and techniques do you use for debugging low-level system code?
How to Answer
- 1
Mention specific debugging tools like GDB or LLDB.
- 2
Explain using printf statements for logging important values.
- 3
Discuss analyzing core dumps for post-mortem debugging.
- 4
Talk about using memory analysis tools like Valgrind.
- 5
Highlight the importance of unit tests and automated testing for early bug detection.
Example Answers
I primarily use GDB for debugging low-level system code, as it allows me to set breakpoints and inspect memory directly. I also add printf statements to check variable values during runtime, which helps track down issues quickly.
Explain how you would optimize a section of code written in C for performance. What techniques would you use?
How to Answer
- 1
Profile the code to find bottlenecks using tools like gprof or Valgrind.
- 2
Examine algorithms and data structures for efficiency improvements.
- 3
Use compiler optimizations and flags during compilation for better performance.
- 4
Reduce memory allocation overheads by reusing allocated memory.
- 5
Apply loop unrolling and vectorization where beneficial to minimize iteration overhead.
Example Answers
I would start by profiling the code to identify slow sections using gprof. Then, I would analyze the algorithms being used and consider replacing any that are inefficient with faster alternatives. Additionally, I would compile the code with optimization flags like -O2 or -O3 to leverage compiler optimizations.
Don't Just Read Systems Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Systems Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What are the main differences between a monolithic kernel and a microkernel? Which do you prefer and why?
How to Answer
- 1
Define both monolithic and microkernel clearly.
- 2
List key differences such as architecture, performance, and modularity.
- 3
State your preference and provide a reason backed by examples.
- 4
Discuss scenarios where each kernel type is advantageous.
- 5
Be prepared to mention real-world operating systems that use each type.
Example Answers
A monolithic kernel includes all operating system services in one large block of code, while a microkernel includes only the most essential services, making it smaller and more modular. I prefer microkernels for their stability and easier maintenance, as the failure of one service doesn't crash the entire system.
How would you use a scripting language to automate common system administration tasks?
How to Answer
- 1
Identify repetitive tasks that take up time.
- 2
Choose a suitable scripting language for the environment, like Python or Bash.
- 3
Write scripts to perform tasks such as backups, user management, or monitoring.
- 4
Test scripts in a safe environment before deployment.
- 5
Implement logging in scripts to track their execution and errors.
Example Answers
To automate backups, I would write a Bash script that uses cron jobs to compress and copy files to a remote server every night, ensuring data safety.
Describe how memory layout is managed in a typical operating system. How do you ensure efficient memory utilization?
How to Answer
- 1
Explain the concept of memory segmentation and paging.
- 2
Discuss the role of the memory management unit (MMU).
- 3
Mention the use of virtual memory and its benefits.
- 4
Talk about techniques like compaction and garbage collection.
- 5
Emphasize the importance of minimizing fragmentation.
Example Answers
In a typical operating system, memory layout is managed through segmentation and paging, allowing for efficient allocation of memory. The MMU translates virtual addresses to physical addresses, enabling processes to use more memory than is physically present through virtual memory techniques. To ensure efficient memory utilization, we minimize fragmentation by using algorithms like best-fit or worst-fit for allocation.
How do low-level system programming tasks interface with network protocols? Give an example of a network-related system programming task.
How to Answer
- 1
Understand how system programming interacts with the OS kernel and networking stacks.
- 2
Focus on memory management, sockets, and device drivers as key areas.
- 3
Provide a clear example that illustrates your understanding of network protocols.
- 4
Mention specific protocols like TCP/IP or UDP when giving examples.
- 5
Explain how low-level coding can affect performance and reliability of network operations.
Example Answers
Low-level system programming tasks like socket programming directly interface with network protocols such as TCP/IP. For example, writing a simple server in C that uses sockets to listen for incoming connections illustrates how we handle network data at the system level.
Describe how a systems programmer interacts with hardware devices at a low level.
How to Answer
- 1
Explain the role of device drivers in communicating with hardware.
- 2
Mention the importance of memory management and addressing in hardware interaction.
- 3
Discuss how interrupt handling works and its significance.
- 4
Highlight the use of system calls for interacting with hardware.
- 5
Provide examples of programming languages or tools commonly used in low-level programming.
Example Answers
A systems programmer interacts with hardware through device drivers, which act as intermediaries. They manage memory to ensure the correct addresses are used. When hardware devices send interrupts, the programmer handles these via interrupt service routines to respond timely. System calls are then used to communicate with the operating system to perform tasks like reading inputs from a keyboard.
What are the challenges of multi-threaded programming? How do you avoid common pitfalls like deadlocks?
How to Answer
- 1
Identify key challenges like race conditions, deadlocks, and complexity.
- 2
Discuss thread safety and data consistency as major concerns.
- 3
Explain synchronization techniques like using mutexes or semaphores.
- 4
Mention the importance of avoiding circular dependencies to prevent deadlocks.
- 5
Suggest using tools like thread analyzers or debuggers to help identify issues.
Example Answers
Multi-threaded programming faces challenges such as race conditions and deadlocks. To avoid these, I ensure proper synchronization with mutexes and design my system to avoid circular dependencies. Additionally, I use thread analyzers to detect potential issues early.
Can you explain the system call mechanism in Unix-like operating systems? How would you implement a new system call?
How to Answer
- 1
Start by defining what a system call is and its role in user-kernel communication.
- 2
Explain the steps involved in making a system call: user mode to kernel mode transition, parameter passing, and returning to user mode.
- 3
Discuss the syscall table and how it maps system call numbers to functions.
- 4
Outline the implementation process for adding a new system call, including kernel modification and recompilation.
- 5
Mention the importance of testing the new system call after implementation.
Example Answers
A system call is an interface that allows user applications to request services from the kernel. When an application makes a system call, it triggers a switch from user mode to kernel mode, allowing the kernel to execute its routines. To implement a new system call, we first define the function prototype, then add it to the syscall table, and finally recompile the kernel. Testing it is crucial to ensure it works as expected.
How do you profile and optimize system performance at the code and execution level?
How to Answer
- 1
Identify bottlenecks using profiling tools like gprof or perf.
- 2
Analyze memory usage and CPU cycles for hotspots in the code.
- 3
Refactor inefficient algorithms or data structures.
- 4
Implement caching mechanisms to reduce repeated computations.
- 5
Test changes with benchmarks to measure performance improvements.
Example Answers
I use profiling tools such as gprof to identify bottlenecks in the application. After pinpointing slow functions, I analyze their memory usage patterns and CPU cycles. If an algorithm proves inefficient, I refactor it to a more optimal one, often introducing caching to avoid redundant computations. Finally, I run benchmarks to ensure the modifications yield significant performance gains.
Don't Just Read Systems Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Systems Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
What role does version control play in systems programming and how do you manage changes across different system components?
How to Answer
- 1
Emphasize the importance of tracking changes and collaboration in development.
- 2
Discuss how version control systems (like Git) help in maintaining history and rollback capabilities.
- 3
Mention branching strategies for managing features or fixes across components.
- 4
Explain how version control aids in continuous integration and deployment workflows.
- 5
Highlight collaboration features that resolve conflicts and coordinate team efforts.
Example Answers
Version control is crucial as it allows us to track changes over time, making it easy to collaborate. For instance, I typically use Git to manage my projects and create branches for new features. This way, I can work on different components without affecting the main codebase until I’m ready to merge.
Explain how a compiler for a systems programming language works at a high level. What optimizations does it perform?
How to Answer
- 1
Begin with the basic stages of a compiler: lexical analysis, syntax analysis, semantic analysis, optimization, and code generation.
- 2
Mention that a compiler translates high-level code into machine code for efficient execution.
- 3
Highlight key optimizations like dead code elimination, loop unrolling, and inlining for performance improvements.
- 4
Avoid deep technical jargon; keep the explanation straightforward and focused on the process.
- 5
Conclude with the benefits of optimizations in terms of speed and resource management.
Example Answers
A compiler for a systems programming language operates through several stages. First, it performs lexical analysis to tokenize source code. Then, it conducts syntax and semantic analysis to ensure correct structure and meaning. After that, the compiler optimizes the intermediate representation, applying techniques like loop unrolling and dead code elimination to enhance performance before generating the final machine code.
Behavioral Interview Questions
Describe a time when you had to troubleshoot a complex system issue. What steps did you take to resolve it?
How to Answer
- 1
Identify the specific system issue you encountered.
- 2
Explain the process you used to diagnose the problem.
- 3
Discuss the steps you took to implement a solution.
- 4
Share the outcome and what you learned from the experience.
- 5
Highlight any tools or methods that were particularly helpful.
Example Answers
In my previous job, we faced a server outage that affected multiple applications. I first checked the server logs for any error messages. After isolating the issue to a memory leak, I used performance monitoring tools to pinpoint the exact process. Once I identified the faulty application, I applied a patch that resolved the memory issue, restoring service within an hour. I learned the importance of thorough log analysis in troubleshooting.
Can you give an example of a successful project you worked on as part of a team? What was your specific role?
How to Answer
- 1
Choose a relevant project that showcases your technical skills and teamwork.
- 2
Briefly outline the project goal and success metrics.
- 3
Clearly define your role and contributions to the team.
- 4
Mention any challenges faced and how the team overcame them.
- 5
Conclude with the outcome and what you learned from the experience.
Example Answers
In my last role, I worked on developing a high-performance file system. The project's goal was to improve read/write speeds by 50%. I was responsible for optimizing the caching algorithms and led a small team to implement these changes. We faced challenges with data consistency, but we overcame them by introducing thorough testing protocols. Ultimately, we increased performance by 60%, which was a great team achievement.
Don't Just Read Systems Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Systems Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Have you ever had to lead a team in a systems programming task? How did you ensure goal alignment and quality output?
How to Answer
- 1
Describe a specific project where you led a team.
- 2
Explain how you aligned team goals with project objectives.
- 3
Mention tools or methods you used to track progress and quality.
- 4
Share how you communicated with your team throughout the process.
- 5
Highlight any outcomes or improvements that resulted from your leadership.
Example Answers
In a recent project, I led a team developing a kernel module. We set clear objectives aligned with the overall system requirements from the start. I used Agile methodologies to track our progress and held daily stand-ups to address any issues promptly. Through regular updates and code reviews, we maintained high quality, resulting in the module being delivered ahead of schedule.
Describe a situation where you disagreed with a team member about a technical solution. How did you handle it?
How to Answer
- 1
Identify the disagreement clearly and concisely
- 2
Explain your reasoning while showing respect for their position
- 3
Discuss how you approached the conversation professionally
- 4
Mention the outcome and any compromises made
- 5
Reflect on what you learned from the situation
Example Answers
In a project, a team member suggested using a specific database technology that I believed wouldn't scale for our needs. I initiated a respectful discussion, presenting my concerns and supporting data. We both explored alternatives, and eventually agreed on a solution that combined elements from both our approaches, which led to successful implementation and better performance.
Tell me about a time when you had to learn a new technology or system quickly. What approach did you take?
How to Answer
- 1
Identify a specific project or challenge where you had to learn quickly.
- 2
Describe the technology or system you learned and why it was important.
- 3
Explain the steps you took to learn it effectively under pressure.
- 4
Highlight any resources you used, such as documentation, online courses, or mentors.
- 5
Conclude with the outcome of your efforts and what you learned from the experience.
Example Answers
In my previous role, I was tasked with implementing a new cloud service within a tight deadline. I quickly familiarized myself with AWS by going through the official documentation and taking an online crash course. I set up a test environment to experiment and get hands-on experience. In just a week, I was able to deploy our application successfully and improve our uptime by 20%.
What is the toughest technical decision you've had to make in your career, and how did you go about making it?
How to Answer
- 1
Identify a specific technical decision that had significant impact.
- 2
Explain the context and the stakes involved in the decision.
- 3
Describe the options you considered and why they were challenging.
- 4
Discuss the process you used to evaluate those options.
- 5
Share the outcome and any lessons learned from the decision.
Example Answers
One of the toughest decisions I faced was whether to rewrite a legacy application in a new language or to maintain it as is. The stakes were high since the application was critical for our operations. I evaluated the pros and cons of both options, consulted with my team, and chose to gradually rewrite it while ensuring we didn't disrupt ongoing operations. Ultimately, we improved performance and maintainability, and I learned the importance of incremental change.
Situational Interview Questions
You are tasked with identifying and fixing a random system crash that occurs under high load. How would you approach this?
How to Answer
- 1
Reproduce the crash under controlled conditions to gather data
- 2
Examine system logs and dump files for errors or patterns
- 3
Use monitoring tools to analyze system performance during high load
- 4
Isolate components of the system to determine which is causing the crash
- 5
Implement fixes based on findings and rigorously test for stability
Example Answers
First, I would try to recreate the crash in a testing environment to observe the behavior under load and gather relevant data. Next, I would check system logs for any error messages or warnings preceding the crash. Monitoring tools like top or vmstat would help me identify resource usage spikes during high load, pinpointing potential bottlenecks.
Your team has been asked to document a large codebase in a short amount of time. How would you organize and approach this task?
How to Answer
- 1
Break down the codebase into manageable modules or components
- 2
Assign documentation tasks based on individual team members' strengths
- 3
Use existing comments and code structure to guide documentation effort
- 4
Establish clear templates for documentation for consistency
- 5
Set deadlines for each module to ensure timely completion
Example Answers
I would start by splitting the codebase into smaller, manageable parts and assign each part to a team member based on their expertise. Then, I would leverage existing comments and structure of the code to create initial documentation, using a consistent template for uniformity.
Don't Just Read Systems Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Systems Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Consider a scenario where system resources are critically low. How would you prioritize and manage processes to restore functionality?
How to Answer
- 1
Identify processes consuming the most resources using monitoring tools.
- 2
Terminate or pause non-essential processes to free up resources.
- 3
Prioritize critical system processes and services that must remain active.
- 4
Implement resource limits or quotas for less critical processes.
- 5
Continuously monitor system performance after adjustments to ensure stability.
Example Answers
First, I would use tools like top or htop to identify processes consuming excessive CPU or memory. Then, I would terminate or pause any non-essential processes. I would ensure critical system services stay active, like databases or web services. Next, I would apply resource limits to less critical processes to balance the load. Throughout this process, I'd monitor system performance to ensure that functionality is restored without causing further issues.
An important system library has a security flaw that needs fixing immediately. What steps will you take to expedite a patch?
How to Answer
- 1
Quickly assess the severity of the flaw and its potential impact.
- 2
Identify the specific components of the library affected by the flaw.
- 3
Communicate with relevant stakeholders and gather a team for a swift response.
- 4
Develop the patch code following security best practices and conduct immediate tests.
- 5
Deploy the patch to production with a rollback plan and monitor closely.
Example Answers
First, I would evaluate how critical the security flaw is and determine its potential risks. Then, I'd identify which parts of the library are affected and gather a small team to work on the patch. I would code the fix, ensuring to follow security protocols, and run quick tests. After that, I would deploy the patch with a clear rollback strategy in case of issues.
You are given a tight deadline for a significant system feature alongside your regular workload. How do you allocate your time and resources?
How to Answer
- 1
Assess the importance and urgency of the feature
- 2
Prioritize tasks based on deadlines and impact
- 3
Break the feature down into smaller, manageable tasks
- 4
Communicate with your team about workload and deadlines
- 5
Consider using time management tools like Gantt charts or Kanban boards
Example Answers
I would first evaluate the urgency of the new feature and see if I can delegate some of my regular tasks. Then, I would break the feature into key milestones to track progress and ensure timely delivery.
How would you plan and execute a complex system upgrade to minimize downtime and user disruption?
How to Answer
- 1
Perform a thorough assessment of the current system architecture.
- 2
Develop a detailed upgrade plan with timelines and milestones.
- 3
Implement a staged upgrade to run in parallel with the existing system.
- 4
Schedule the upgrade during off-peak hours to reduce user impact.
- 5
Test the upgrade in a sandbox environment before actual execution.
Example Answers
I would start by assessing the current system to identify dependencies. Then, I'd create a plan that includes a timeline with milestones. During the execution, I'd run the upgrade in stages, allowing both systems to operate simultaneously. Scheduling this during off-peak hours would help minimize user disruption, and finally, I’d test everything in a controlled environment beforehand.
A critical system component you developed is failing intermittently, and management needs a briefing. How do you explain the issue and solution?
How to Answer
- 1
Start with a brief overview of the component and its importance.
- 2
Explain the nature of the failure clearly and concisely.
- 3
Outline the steps taken to diagnose the problem.
- 4
Present the proposed solution along with its benefits.
- 5
Be prepared to answer any follow-up questions regarding risks and timelines.
Example Answers
The critical component is our data processing module, vital for real-time analytics. We've noticed it fails intermittently during peak load. After investigation, we found it was due to a memory leak. My proposed solution is to optimize memory usage and implement additional caching. This will improve stability and performance during high-demand periods.
You've proposed a major change to the system architecture to improve efficiency. How do you gain buy-in from stakeholders and ensure a smooth transition?
How to Answer
- 1
Identify key stakeholders and their concerns early on
- 2
Create a clear, data-driven presentation of benefits and risks
- 3
Engage stakeholders in discussions to address their questions
- 4
Provide a detailed implementation plan with timelines
- 5
Offer training and support to ease the transition
Example Answers
First, I would identify and understand the concerns of key stakeholders, like the project managers and the development team. Then, I'd prepare a clear presentation showing the benefits of the proposed system architecture change, supported by data. I would schedule a meeting to engage them, allowing for open discussion. I would present a detailed implementation plan with specific timelines and assure them that training and support will be provided during the transition to minimize disruptions.
A project you are leading is behind schedule due to unforeseen technical challenges. How do you address this with your team and stakeholders?
How to Answer
- 1
Acknowledge the issue clearly and honestly with the team.
- 2
Gather data on the current status and specific challenges.
- 3
Develop a revised plan with input from the team.
- 4
Communicate the revised plan to stakeholders promptly.
- 5
Monitor progress closely to adjust strategies as needed.
Example Answers
I would first hold a meeting with the team to openly discuss the challenges we are facing. I would ensure everyone understands the current status and gather input on how we can overcome these hurdles. Then, I'd create a new timeline and present this to our stakeholders, explaining the necessary adjustments.
You're tasked with making an existing system application cross-platform. What challenges do you anticipate, and how do you tackle them?
How to Answer
- 1
Identify platform-specific dependencies such as libraries and APIs
- 2
Consider user interface design differences across platforms
- 3
Evaluate performance implications on different operating systems
- 4
Use cross-platform frameworks or tools where applicable
- 5
Plan thorough testing on all targeted platforms to ensure functionality
Example Answers
I anticipate challenges with platform-specific libraries and APIs. To tackle this, I would identify these dependencies early and either find cross-platform alternatives or abstract them in a way that allows for different implementations on each platform.
Don't Just Read Systems Programmer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Systems Programmer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
A large client reports frequent system slowdowns. You believe it's due to their setup. How do you address their concerns diplomatically and technically?
How to Answer
- 1
Acknowledge the client's concerns to show understanding.
- 2
Ask for specific details about their experiences with the slowdowns.
- 3
Suggest a collaborative investigation into possible causes of the issue.
- 4
Present potential solutions or adjustments based on your findings.
- 5
Reassure them of your commitment to resolving the issue promptly.
Example Answers
I understand that system slowdowns can be frustrating. Could you provide me with some specific examples of when these slowdowns occur? I believe we can work together to analyze their setup and identify potential improvements that can enhance performance. I'm committed to finding a solution as quickly as possible.
Systems Programmer Position Details
Salary Information
Recommended Job Boards
These job boards are ranked by relevance for this position.
Related Positions
- Software Programmer
- Database Programmer
- Application Programmer
- Game Programmer
- Computer Programmer
- Programmer
- Website Programmer
- Web Programmer
- .NET Programmer
- Java Programmer
Similar positions you might be interested in.
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates