Top 30 Python Developer Interview Questions and Answers [Updated 2025]
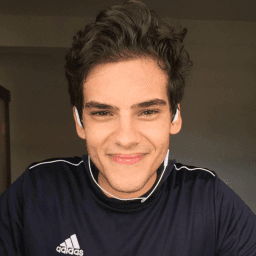
Andre Mendes
•
March 30, 2025
Navigating the competitive landscape of Python development requires preparation and insight. In this post, we delve into the most common interview questions asked for the 'Python Developer' role, providing not only example answers, but also expert tips on crafting effective responses. Whether you're a seasoned coder or just starting out, this guide will help you stand out in your next interview.
Download Python Developer Interview Questions in PDF
To make your preparation even more convenient, we've compiled all these top Python Developerinterview questions and answers into a handy PDF.
Click the button below to download the PDF and have easy access to these essential questions anytime, anywhere:
List of Python Developer Interview Questions
Technical Interview Questions
What are Python decorators and how do you use them?
How to Answer
- 1
Define what a decorator is: a function that modifies another function.
- 2
Explain the syntax using the '@' symbol.
- 3
Provide a simple example to illustrate usage.
- 4
Mention use cases, like logging or access control.
- 5
Keep it clear and concise, avoid technical jargon.
Example Answers
Python decorators are functions that take another function and extend its behavior without modifying it. You use them with the '@' symbol above the function definition. For example, if you have a function 'my_function', you can create a decorator 'my_decorator' and use it like this: '@my_decorator'. This is commonly used for logging or enforcing access controls.
Explain the difference between a list and a tuple in Python.
How to Answer
- 1
State that lists are mutable and tuples are immutable.
- 2
Mention that lists use square brackets and tuples use parentheses.
- 3
Highlight that lists have more built-in methods compared to tuples.
- 4
Explain that tuples can be used as keys in dictionaries, while lists cannot.
- 5
Provide a use-case example for each type.
Example Answers
Lists are mutable, meaning you can change their content, while tuples are immutable, so they can't be altered after creation. Lists are defined with square brackets, like [1, 2, 3], whereas tuples are defined with parentheses, like (1, 2, 3). Lists have more methods available, such as append and extend. You can use tuples as dictionary keys because they are immutable, while lists cannot be used for that purpose. For example, I would use a list to hold a collection of user inputs, and a tuple to hold a fixed set of coordinates.
Don't Just Read Python Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Python Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
How can you optimize the performance of a Python script?
How to Answer
- 1
Identify bottlenecks using profiling tools like cProfile
- 2
Use built-in functions and libraries that are optimized for performance
- 3
Avoid excessive memory usage by using generators instead of lists
- 4
Utilize multiprocessing or multithreading for CPU-bound or I/O-bound tasks
- 5
Optimize algorithms and data structures for efficiency
Example Answers
One way to optimize a Python script is by profiling it with cProfile to find bottlenecks, then replace any slow parts with faster built-in functions. For example, using sum() instead of a loop to sum elements can greatly improve speed.
What is the @staticmethod decorator in Python, and when would you use it?
How to Answer
- 1
Define @staticmethod and its purpose.
- 2
Explain that it allows a method to be called on a class without reference to an instance.
- 3
Mention when you might use it, such as when the method is logically related to the class but doesn't need access to class or instance data.
- 4
Provide a simple example to illustrate its usage.
- 5
Conclude with a note on readability and organization in class design.
Example Answers
The @staticmethod decorator defines a method that belongs to the class rather than any instance of the class. You use it when the method does not require access to instance-specific data, such as utility functions related to class functionality.
What are some Python libraries you have used for data analysis and what are their strengths?
How to Answer
- 1
Identify 3 to 4 libraries relevant to data analysis in Python.
- 2
For each library, explain its primary use case and strength.
- 3
Mention any personal experience or projects where you used these libraries.
- 4
Be specific about what you accomplished with each library.
- 5
Keep your answers concise and focused on practical applications.
Example Answers
I have used Pandas for data manipulation and cleaning, which is great for handling large datasets. I utilized it in a project analyzing sales data to identify trends.
How does exception handling work in Python? Can you give me an example of how to use try/except blocks?
How to Answer
- 1
Explain that exceptions are errors that can be caught and handled.
- 2
Mention the use of try to execute code that might raise an exception.
- 3
Describe how except can catch specific exceptions or a generic exception.
- 4
Highlight the optional else block for code that runs if no exception occurs.
- 5
Include a finally block that executes regardless of whether an exception occurred.
Example Answers
In Python, exception handling allows us to manage errors gracefully. We use try to enclose code that might raise an error. For example: try: print(1/0) except ZeroDivisionError: print('Cannot divide by zero'). The except block handles the error when it occurs.
What testing frameworks have you used in Python, and how do you implement unit tests?
How to Answer
- 1
Mention specific frameworks like unittest, pytest, or doctest.
- 2
Explain a simple structure of a unit test including setup and assertions.
- 3
Provide an example of a test case you implemented.
- 4
Discuss how you ensure tests are isolated and maintainable.
- 5
Highlight any CI/CD practices you follow for running tests.
Example Answers
I have used unittest and pytest extensively. For instance, in pytest, I define a test function that begins with 'test_', and use assertions to check expected outcomes. I also ensure each test is independent by using fixtures for setup.
Can you explain what metaclasses are in Python, and provide an example use case?
How to Answer
- 1
Define what a metaclass is in Python clearly and simply
- 2
Explain how metaclasses modify class creation
- 3
Give a practical example of when you might use a metaclass
- 4
Mention how metaclasses relate to the type function
- 5
Keep the explanation concise; avoid overly technical jargon
Example Answers
Metaclasses are classes of classes; they define how a class behaves. In Python, everything is an object, including classes themselves. They allow you to customize class creation. For instance, a common use case is enforcing coding standards or automatically registering classes in a framework.
How have you used Python for web development in the past? Which frameworks do you prefer?
How to Answer
- 1
Start by mentioning specific projects you've worked on using Python for web development.
- 2
Highlight the frameworks you've used, such as Django or Flask, and why you prefer them.
- 3
Discuss any tools or libraries that complemented your web development work in Python.
- 4
Share your experience regarding deployment, if applicable.
- 5
Keep your response concise and related to your relevant work experience.
Example Answers
In my last project, I developed a RESTful API using Flask, which interfaced with a React frontend. I prefer Flask for its simplicity and clarity, especially for small to medium-sized applications.
How do you typically integrate version control with Python development? Can you describe your workflow?
How to Answer
- 1
Start by explaining your choice of version control system, such as Git.
- 2
Outline your branching strategy, like using feature branches for new development.
- 3
Mention how you commit your changes frequently with clear messages.
- 4
Discuss your process for merging changes back to the main branch, including code reviews.
- 5
Conclude with how you handle deployment and versioning for releases.
Example Answers
I use Git as my version control system. I typically create a new branch for each feature I work on, committing changes frequently with descriptive messages. Once the feature is complete, I open a pull request for a code review, and merge back into the main branch after approval. For releases, I tag the commits with version numbers.
Don't Just Read Python Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Python Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Behavioral Interview Questions
Can you describe a time when you had to work closely with a team to complete a project using Python?
How to Answer
- 1
Choose a specific project that involved significant collaboration.
- 2
Highlight your role and contributions in the team.
- 3
Explain tools and technologies used in the project.
- 4
Mention how collaboration was structured (meetings, code reviews, etc.).
- 5
Discuss the outcome and what you learned from the experience.
Example Answers
At my last job, our team developed a web application using Flask. I was responsible for the backend API and worked closely with front-end developers. We held daily stand-up meetings and utilized Git for version control, ensuring smooth collaboration. This experience taught me the importance of clear communication and the role of documentation in team projects.
Tell me about a time when you disagreed with a team member on how to implement a feature in Python. How did you handle it?
How to Answer
- 1
Describe the situation briefly and who was involved
- 2
Explain the differing opinions on the implementation
- 3
Focus on communication: how you discussed your views
- 4
Highlight the outcome and any compromises made
- 5
Reflect on what you learned from the experience
Example Answers
In my previous project, I disagreed with a team member about whether to use a synchronous or asynchronous approach for a data processing feature. I believed that using async would make the app more responsive. We had a meeting where I presented my case with performance metrics, and he shared his concerns about complexity. We eventually agreed on a compromise where we implemented a basic version sync first, and then planned to refactor to async later if needed. This taught me the value of clear communication and iteration.
Don't Just Read Python Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Python Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Describe a challenging problem you encountered while programming in Python and how you solved it.
How to Answer
- 1
Choose a specific problem that highlights your skills.
- 2
Explain the context and why it was challenging.
- 3
Detail the steps you took to analyze and solve the problem.
- 4
Mention any tools or libraries you used in your solution.
- 5
Conclude with the outcome and what you learned.
Example Answers
I faced a performance issue with a data processing script that was taking too long. I analyzed the code and figured out that nested loops were slowing it down. I replaced them with a more efficient algorithm using sets for lookups. This reduced the processing time from several minutes to just a few seconds, and I learned a lot about algorithm efficiency.
Give an example of a project where you had to learn a new Python library or framework quickly. How did you approach learning it?
How to Answer
- 1
Identify the project and the library you needed to learn.
- 2
Explain the urgency or reason for learning quickly.
- 3
Describe specific resources you used to learn the library.
- 4
Mention how you applied the library in your project.
- 5
Highlight any challenges you faced and how you overcame them.
Example Answers
In my previous job, I was tasked with building a web application using Flask on short notice. I quickly checked the Flask documentation and followed a few online tutorials. I set up a minimal example and gradually integrated the main features I needed. One challenge was understanding how routing worked, but I used the Flask documentation to clarify that.
Tell me about a time you managed multiple Python projects simultaneously. How did you prioritize and manage them?
How to Answer
- 1
Identify your most critical project and its deadlines.
- 2
Use a task management tool to keep track of progress.
- 3
Set aside dedicated times for each project to ensure focus.
- 4
Communicate regularly with stakeholders on project statuses.
- 5
Be flexible and ready to adjust priorities as needs change.
Example Answers
In my last job, I was juggling three Python projects. I prioritized them based on deadlines and the impact on the company. I used Trello to manage tasks and set aside dedicated work blocks for each project. Regular updates kept everyone aligned, and I was able to deliver on time.
Can you describe a time when you took the initiative to improve an existing Python script or process?
How to Answer
- 1
Choose a specific instance where you noticed a problem.
- 2
Explain the impact of the existing script on the team or project.
- 3
Describe the changes you implemented in the script.
- 4
Quantify the results of your improvements when possible.
- 5
Highlight any skills or tools you used during the process.
Example Answers
In my previous role, I noticed our data processing script was taking too long to complete. I analyzed the code and identified redundant loops. I refactored it using list comprehensions, which reduced the processing time by 50%. This allowed the team to receive results sooner and improved overall productivity.
What is the most complex Python project you have worked on, and what did you learn from that experience?
How to Answer
- 1
Choose a project that showcases your skills and challenges faced
- 2
Highlight the specific technologies and libraries you used
- 3
Explain your role in the project and any leadership taken
- 4
Discuss the challenges and how you overcame them
- 5
Reflect on the key lessons learned and how they apply to future work
Example Answers
In my last role, I led a team to build a Django web application for real-time data processing. We used Celery for task queues and PostgreSQL as our database. The biggest challenge was ensuring data integrity during high loads. I learned the importance of optimizing database queries and how to manage asynchronous tasks effectively.
Describe a situation where you had to meet a tight deadline with a Python project. How did you manage your time?
How to Answer
- 1
Identify a specific project and deadline.
- 2
Explain the importance of the project to stakeholders.
- 3
Outline steps taken to prioritize tasks efficiently.
- 4
Discuss any tools or techniques used to stay organized.
- 5
Reflect on what you learned from the experience.
Example Answers
In my last role, I had a project to deliver a data analysis tool using Python within one week. I prioritized key features to build a minimum viable product. I used Trello to track tasks and assigned deadlines for each. By focusing on the core functionality first, I delivered on time and received positive feedback.
Tell me about a time you used Python to develop an innovative solution to a problem.
How to Answer
- 1
Identify a specific problem you faced that required innovation.
- 2
Describe how you approached the problem using Python.
- 3
Explain the solution you implemented and why it was innovative.
- 4
Highlight the impact of your solution on the project or team.
- 5
Be ready to discuss any challenges you overcame during the process.
Example Answers
In my previous role, we had inefficiencies in data processing. I used Python with Pandas to develop a script that automated data cleaning, reducing processing time by 50%. This innovation allowed the team to focus on analysis rather than cleanup.
Don't Just Read Python Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Python Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Situational Interview Questions
You're given a Python script that processes a large dataset and it runs slowly. What steps would you take to improve its performance?
How to Answer
- 1
Profile the script to identify performance bottlenecks using tools like cProfile or timeit.
- 2
Optimize algorithms and data structures, choosing more efficient ones where applicable.
- 3
Utilize built-in functions and libraries like NumPy which are optimized for performance.
- 4
Consider parallel processing or multi-threading to make use of multiple CPU cores.
- 5
Reduce memory usage by using generators instead of lists when possible.
Example Answers
First, I would use cProfile to profile the script and find where the slowdown occurs. Then, I'd look for opportunities to optimize the algorithms or data structures being used. If there are any heavy computations, I might switch to NumPy for array operations.
A critical bug has been reported in production for a Python application you maintain. How would you handle investigating and fixing this issue?
How to Answer
- 1
Quickly gather information about the bug from users or logs.
- 2
Reproduce the issue in a development environment using the same conditions.
- 3
Review the relevant code and check recent changes that might relate to the bug.
- 4
Implement a fix and test it thoroughly to ensure it resolves the issue without introducing new ones.
- 5
Deploy the fix to production and monitor the application for any further issues.
Example Answers
First, I would check the logs to gather as much context as possible about the bug. Then, I would try to reproduce the issue in a local environment. After that, I would look at the recent commits to see if any changes are related. Once I identify the root cause, I would implement a fix, test it, and deploy it to production. Finally, I would monitor the application to ensure stability.
Don't Just Read Python Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Python Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
You are the lead Python developer of a project. How would you approach mentoring a junior developer who is struggling with understanding complex code?
How to Answer
- 1
Analyze their current understanding and identify gaps
- 2
Break down the complex code into smaller, manageable pieces
- 3
Use examples to illustrate key concepts clearly
- 4
Encourage open questions and create a safe space for learning
- 5
Schedule regular check-ins to monitor progress and provide feedback
Example Answers
I would first assess what specific areas the junior developer finds confusing. From there, I would break down the code into smaller chunks and explain each part with simple examples, ensuring they understand before moving on. Regular check-ins would help track their progress and reinforce learning.
You have to choose between two Python frameworks for a project. What factors would influence your decision, and how would you make a choice?
How to Answer
- 1
Evaluate the project requirements and goals first
- 2
Consider the learning curve and team expertise with each framework
- 3
Assess performance and scalability needs for the application
- 4
Check community support and available documentation
- 5
Review third-party packages and integrations offered by each framework
Example Answers
I would assess the project requirements and choose the framework that best fits them. If one framework has a steeper learning curve but offers better scalability, I would consider my team's familiarity with it.
You notice a teammate submitted Python code with a significant design flaw. How would you address this in a code review?
How to Answer
- 1
Start with positive feedback about the code.
- 2
Explain the design flaw clearly and its impact.
- 3
Suggest specific changes or improvements.
- 4
Encourage discussion and invite their perspective.
- 5
Keep the tone collaborative and supportive.
Example Answers
I would begin by acknowledging the effort put into the code. Then, I would point out the design flaw, explaining how it could lead to performance issues. I'd suggest an alternative approach that enhances readability and efficiency. Finally, I'd ask for my teammate's thoughts on my suggestions.
A cross-functional team needs to integrate a new Python-based feature with another system. How would you facilitate this integration?
How to Answer
- 1
Identify the APIs or data formats used by both systems.
- 2
Communicate with team members to understand the requirements and constraints.
- 3
Set up a testing environment to prototype the integration.
- 4
Document the integration steps and any dependencies clearly.
- 5
Ensure ongoing collaboration through regular updates and feedback loops.
Example Answers
First, I would analyze the APIs or data formats of both systems to identify how they can communicate. Then, I'd gather inputs from the team to clarify requirements. I would create a prototype in a testing environment and document the integration process. Finally, I'd maintain communication with the team to address any challenges.
A client requests a feature that requires Python programming, but is not feasible due to technical limitations. How would you communicate this to the client?
How to Answer
- 1
Acknowledge the client's request and its importance
- 2
Clearly explain the technical limitations without jargon
- 3
Suggest alternative solutions or workarounds if possible
- 4
Keep the communication positive and collaborative
- 5
Offer to discuss further or explore other feature options
Example Answers
I appreciate your interest in this feature. Unfortunately, due to technical constraints, we can't implement it as requested. However, I can suggest an alternative approach that achieves similar results. Let's discuss this further to see how we can meet your needs.
You are nearing a project deadline, but there are still several untested features in your Python code. How do you handle this situation?
How to Answer
- 1
Prioritize features based on their impact on the project.
- 2
Allocate time for testing the most critical features first.
- 3
Communicate with your team or stakeholders about the situation.
- 4
Consider writing quick integration tests for high-risk areas.
- 5
Maintain documentation of any untested features for future reference.
Example Answers
I would first prioritize the untested features to identify which ones are critical for the project. Then, I would focus on testing those features thoroughly, while communicating any potential risks to my team.
You've been asked to refactor an old Python codebase that lacks documentation. What steps would you take to approach this task?
How to Answer
- 1
Start by running the code to understand its functionality.
- 2
Identify key components and create a high-level architecture diagram.
- 3
Write inline comments while you analyze the code for clarity.
- 4
Refactor smaller functions or classes incrementally, testing as you go.
- 5
Document the code structure and usage in a README or similar file.
Example Answers
I would first run the code to see what it does and get an overview of its functionality. Then, I'd sketch a high-level architecture to identify important components. While analyzing, I'd add inline comments to clarify complex sections. I'd tackle the refactoring in small pieces and document everything in a README.
You are responsible for ensuring the security of a Python application. How would you go about identifying potential security vulnerabilities?
How to Answer
- 1
Conduct a code review focusing on security best practices.
- 2
Use automated tools like Bandit or Snyk to scan for vulnerabilities.
- 3
Implement dependency checking to identify known issues in libraries.
- 4
Perform manual testing such as SQL injection and other attack simulations.
- 5
Stay updated on common vulnerabilities from OWASP and apply necessary fixes.
Example Answers
I would start with a code review, checking for insecure coding practices. Then, I'd use tools like Bandit to scan for vulnerabilities in the codebase. Additionally, I'd verify that all dependencies are up to date and free from known vulnerabilities. Performing some manual tests, like SQL injection, would also be essential.
Don't Just Read Python Developer Questions - Practice Answering Them!
Reading helps, but actual practice is what gets you hired. Our AI feedback system helps you improve your Python Developer interview answers in real-time.
Personalized feedback
Unlimited practice
Used by hundreds of successful candidates
Python Developer Position Details
Salary Information
Recommended Job Boards
These job boards are ranked by relevance for this position.
Related Positions
- JavaScript Developer
- Application Developer
- Software Developer
- C Developer
- Android Developer
- Database Developer
- Software Engineer
- .NET Developer
- Game Developer
- Java Software Engineer
Similar positions you might be interested in.
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates
Ace Your Next Interview!
Practice with AI feedback & get hired faster
Personalized feedback
Used by hundreds of successful candidates