Top 31 Spring Layer Interview Questions and Answers [Updated 2025]
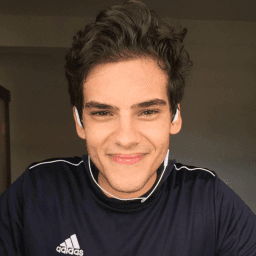
Andre Mendes
•
March 30, 2025
Are you preparing for a Spring Layer role interview and want to maximize your chances of success? This blog post is your ultimate guide, featuring the most common interview questions tailored for this position. We provide not only example answers but also insightful tips on how to respond effectively, ensuring you're well-equipped to impress your interviewers. Dive in and elevate your interview skills today!
Get Spring Layer Interview Questions PDF
Get instant access to all these Spring Layer interview questions and expert answers in a convenient PDF format. Perfect for offline study and interview preparation.
Enter your email below to receive the PDF instantly: